Are You Ignoring these Essential Node.js Practices? Don't Get Left Behind!
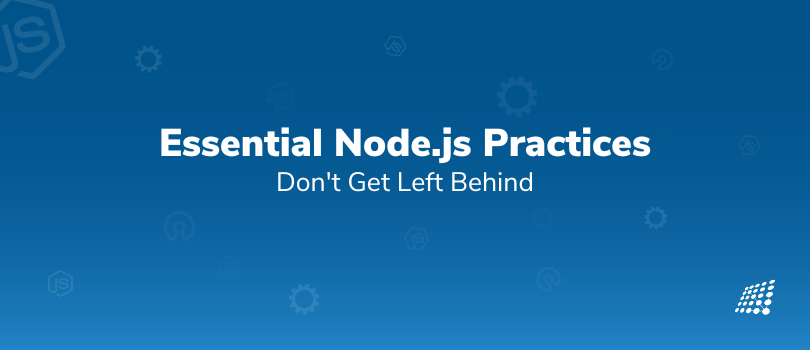
Node.js is turning out to be the superhero of web development! Did you know that Netflix, Amazon, LinkedIn, PayPal, Reddit, and Tumblr all utilize Node.js? Its futuristic features caught the attention of Amazon. Even PayPal and Netflix found that it minimized their startup time from 40+ minutes to under a minute. So it’s no surprise that Node.js features are taking the web development world by storm.
Additionally, Node.js is the most extensively used web development tool in the US, with a staggering 6.3 million websites utilizing its powerful capabilities! Don't miss out on this revolutionary tool that's changing the game for developers everywhere. With it, you can effortlessly build fast and scalable applications.
However, there's also a lot to learn about this platform. Ask yourself, "How aware are you of Node.js’ best practices that help you create efficient and secure apps?" In this blog, we reveal what is Node.js, what is Node.js used for, and the industry's top best practices for mastering Node.js development.
So first! Let’s get down to the basics.
What is Node.js?
Before we get to what is Node.js used for, let’s talk about what is Node.js!
Node.js is a well-known open-source, cross-platform JavaScript runtime environment that allows developers to build server-side and networking applications using JavaScript. It executes JavaScript code outside of a web browser, has a large and active community, and is used by many companies and organizations all over the globe.
To name a few Node.js features, it is lightweight and efficient, built on Chrome's V8 JavaScript engine, and provides an event-driven, non-blocking I/O model to developers. This is the major reason why Node.js is popular for building real-time, data-intensive applications.
When looking at what is Node.js used for, you will see that it is mostly utilized for creating backend services, such as microservices and RESTful APIs. Node.js has a large and active Node.js developer community—the extensive ecosystem of open-source packages and modules known as npm (Node Package Manager). It can be simply installed and utilized in a Node.js application. Developers can easily add functionality to their applications since they do not have to start from scratch. Furthermore, Node.js may be used to deploy apps across a broad range of platforms, as it is compatible with Windows, Linux, and macOS.
The Unique Features that Make Node.js Stand Out?
There are many Node.js best practices you can benefit from, but now let’s look at some of the Node.js features that make it stand out successfully!
JavaScript on the server-side
As we mentioned earlier in the ‘what is Node.js’ section, Node.js is an open-source, cross-platform JavaScript runtime environment that allows developers to run JavaScript on the server side. This means that you can use the same language for both front-end and back-end development. This automatically makes processes and proceedings a whole lot simpler for developers.
Non-blocking I/O model
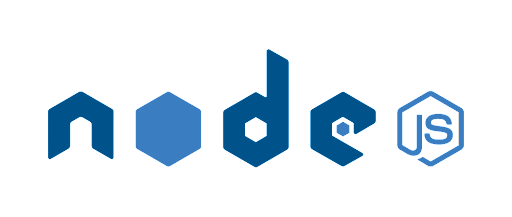
One of the aspects that makes Node.js special is its non-blocking I/O model. This means that it's lightweight and efficient, making it ideal for building real-time, data-intensive applications like chat apps, live-streaming platforms, and online games.
Package Manager
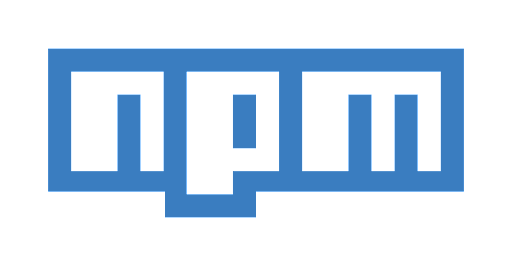
Among the other Node.js features that are pretty cool is its package manager, npm. This allows developers to easily install and manage third-party libraries and modules, saving them a ton of time and effort. It's like having a superpower for developers.
Large developer community
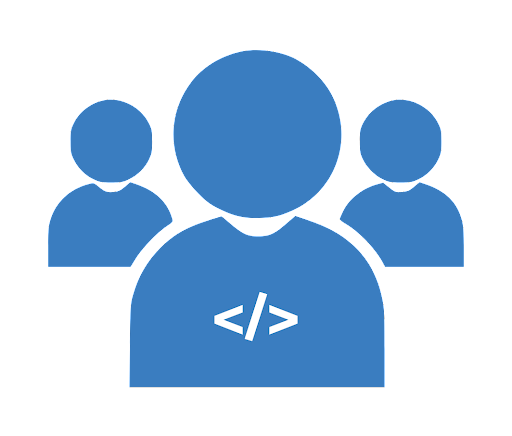
Node.js also has a huge and active developer community, which has led to the creation of a vast ecosystem of open-source packages and libraries. This makes it easy for developers to find solutions to common problems and add functionality to their applications without having to start from scratch.
Cross-platform Compatibility
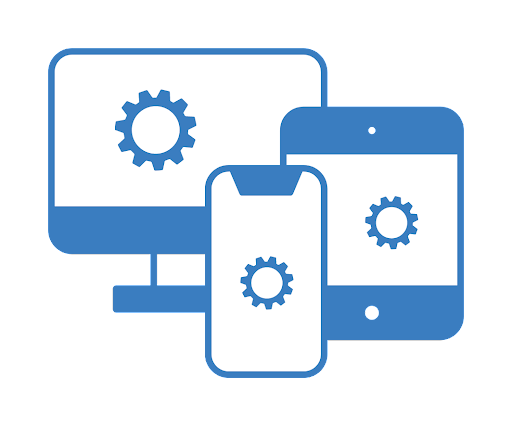
And perhaps the best part? Node.js is compatible with various operating systems, including Windows, Linux, and macOS, which makes it easy to deploy applications on different environments.
High-performance
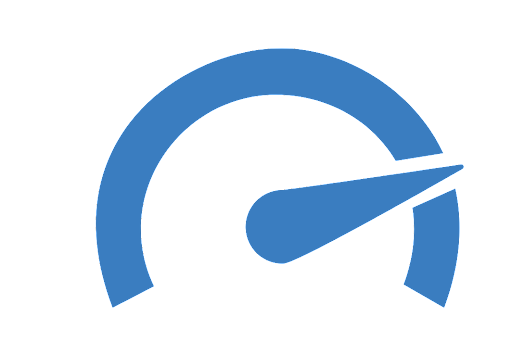
Node.js is a flexible, high-performance, and efficient runtime environment that can be used to build a wide range of applications, from web apps to IoT devices, and with the help of its package manager and developer community, can make the development process much easier and faster.
Flexibility
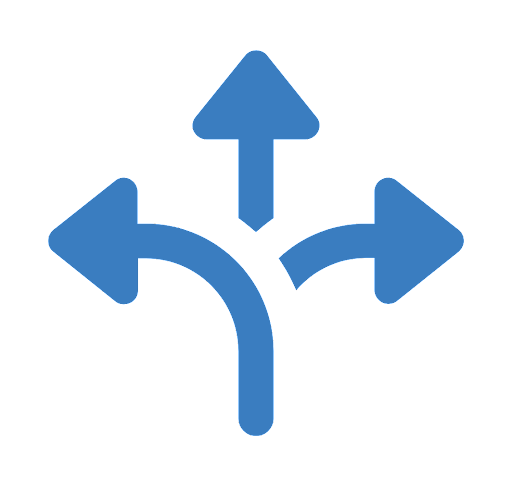
Node.js is flexible and can be used for a wide range of applications, from web applications and mobile apps to IoT devices and robots. This is the reason why the best practices for Node.js can be skillfully put to the test.
Node.js Best Practices to Follow
There are a few Node.js best practices that you should follow when developing applications. These include:
- Use async/await for asynchronous code: Async/await makes it easier to write asynchronous code that is easy to read and debug.
async function getData() {
try {
const response = await fetch('https://my-api.com/endpoint');
const data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
- Use error-first callbacks: When writing callback functions, it is good practice to pass the error as the first argument. This makes it easier to handle errors and ensures that the callback is only called when there is no error.
fs.readFile('/path/to/file', (error, data) => {
if (error) {
console.error(error);
return;
}
console.log(data);
});
- Use the Node.js debugger: The Node.js debugger is a powerful tool for debugging your code. It allows you to set breakpoints, step through code, and inspect variables.
Example:
To start the debugger, you can use the debug command followed by the name of your script:
node debug my-script.js
- Use linting tools: Linting tools, such as ESLint, can help to enforce coding standards and catch common mistakes.
Example:
To install and configure ESLint, you can use the following commands:
npm install -g eslint
eslint --init
- Use modern JavaScript features: If you’re wondering what is Node.js used for, Node.js supports many modern JavaScript features, such as async/await and the spread operator. These features can make your code more concise and easier to understand.
const numbers = [1, 2, 3];
const sum = numbers.reduce((total, number) => total + number, 0);
console.log(sum); // 6
- Use a testing framework: A testing framework, such as Jest or Mocha, can help you write reliable tests for your code.
Example:
Here is an example of a test written using Jest:
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
- Use a code formatter: A code formatter, such as Prettier, can help to ensure that your code is consistently formatted and easy to read.
Example:
To format your code with Prettier, you can use the following command:
prettier --write "**/*.{js,json,css}"
- Use a package manager: A package manager, such as npm or yarn, can help you manage your dependencies and keep them up to date.
Example:
To install a package with npm, you can use the following command:
npm install <package-name>
- Use the util.promisify function to convert callback-based functions to Promises: The util.promisify function allows you to convert a callback-based function to a Promise-based function. This can make it easier to use async/await with functions that don't natively return Promises. Here's an example of how to use util.promisify:
const util = require('util');
const fs = require('fs');
const readFile = util.promisify(fs.readFile);
async function main() {
const data = await readFile('file.txt', 'utf8');
console.log(data);
}
main();
- Use the stream.pipeline function to simplify stream processing: Another among the best practices Node.js is the stream.pipeline. With the stream.pipeline function, you can chain together a series of stream operations, making it easier to write stream processing code. Here's an example of how to use stream.pipeline to copy a file:
const fs = require('fs'); const stream = require('stream');
const pipeline = util.promisify(stream.pipeline);
async function copyFile(src, dest) { await pipeline( fs.createReadStream(src), fs.createWriteStream(dest) ); }
copyFile('src.txt', 'dest.txt');
In conclusion, following best practices when working with Node.js can help you write high-quality, maintainable code that is easy to understand and debug.
Knowing the best practices for Node.js development can make it seamless to write more efficient, maintainable, and scalable code.
By adopting these Node.js best practices, you can improve the reliability and performance of your Node.js applications, and you can make it easier for other developers to collaborate on your code. Whether you are a beginner or an experienced developer, following these best practices for Node.js can help you become more productive and effective when working with this platform.
Ready to take your Node.js development to the next level with a complete MERN stack solution? Our MERN service can help you create high-performing web applications with ease. And to ensure your success, we recommend implementing the best practices for Node.js development. Check out our blog for expert insights and actionable tips. Click here to learn more about our MERN service and read the blog now!
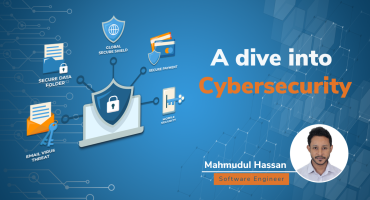
A Dive into Cybersecurity
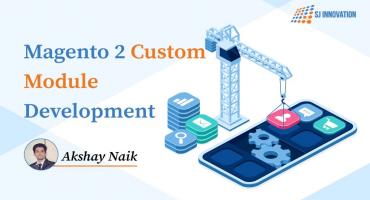
Magento 2 Custom Module Development
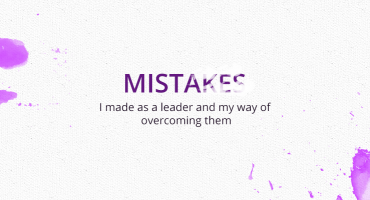