How To Build A Simple Productivity Application With ReactJs
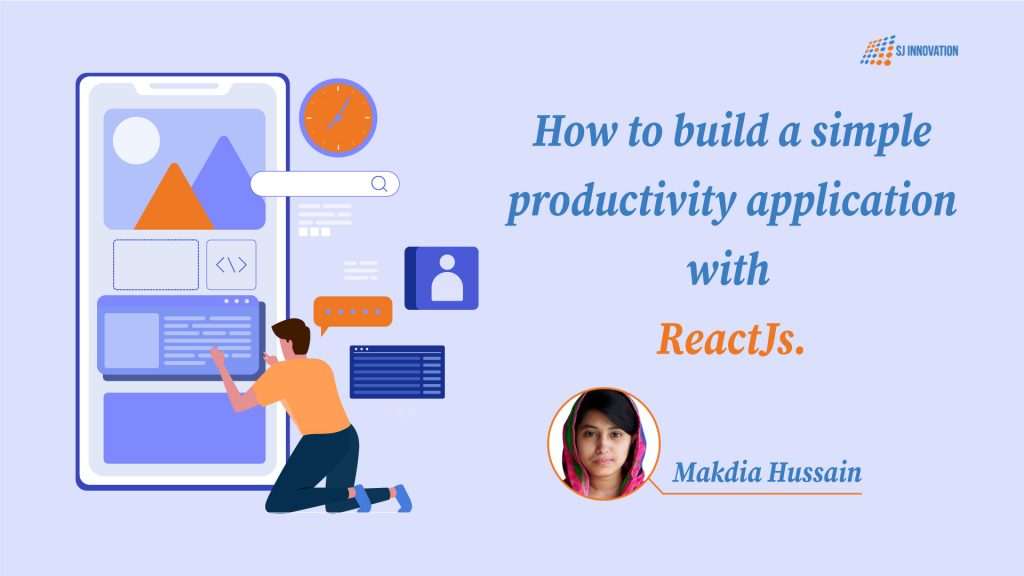
In this article, you will learn how to build a simple productivity application with ReactJs.
ReactJs is a front-end JavaScript library that is used to develop interactive user interfaces. It is maintained by Facebook and a community of individual developers and companies. It is mainly used for single page web or mobile applications. It allows us to create reusable UI components. So in this article, I am choosing the To-Do List App as a productive app. Here you will learn how to create a simple To-Do List app built with React.js. I think building a To-Do List app can be a great way to learn the React technology.
Built Using
- React.js
- React-Router
- Node
Getting Started
Start your project by following the steps below:
Step 1: Starting a React App
- The first step is to create a new React app. From your command line, navigate to the folder you want to create your new project in, and enter the command: npx create-react-app todolist
- Now go to the folder: cd todolist
- Run the project: npm start
- To run the project on the browser, just run the above command. It will run the project on the browser at http://localhost:3000
Step 2: Styling Your Application
Open App.css in your code editor and place the code below:
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
body {
background-color: #EDF1F3;
font-family: Arial, Helvetica, sans-serif;
line-height: 1.4;
}
a {
color: #333;
text-decoration: none;
}
.container {
padding: 0;
margin-bottom: 15px;
background-color: #fff;
border-radius: 0px 0px 5px 5px;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19);
}
button {
border: #7D552D !important;
background: #B07840 !important;
}
.btn {
display: inline-block;
border: #7D552D !important;
background: #B07840 !important;
color: #fff;
padding: 7px 20px;
cursor: pointer;
}
button:hover, .btn:hover {
background-color: #7D552D !important;
border-color: #606c76 !important;
color: #fff !important;
}
form {
padding: 8px 1rem 0 !important;
margin-bottom: 1.5rem !important;
}
input[type="text"] {
border: 0.1rem solid #7D552D !important;
}
p {
margin-top: 1.5rem !important;
margin-bottom: 2rem !important;
}
Step 3 — Fetching To-Do Items
To display the To-Do List items, open src/components/App.js and place the code below:
class App extends Component {
state = {
todos: [
{
"id": 1,
"note": "Design the solution",
"completed": false
},
{
"id": 2,
"note": "Prepare for implementation",
"completed": false
},
{
"id": 3,
"note": "Prepare the test/QA environment",
"completed": false
},
{
"id": 4,
"note": "Install the product in the test/QA environment",
"completed": false
},
{
"id": 5,
"note": "Prepare the production environment",
"completed": false
},
]
}
render() {
return (
<Router>
<div className="App">
<div className="container">
<Header />
<br />
<Route exact path="/" render={props => (
<React.Fragment>
<Todos todos={this.state.todos} markComplete = {this.markComplete} delTodo={this.delTodo}/>
</React.Fragment>
)} />
</div>
</div>
</Router>
);
}
}
Step 4 — AddingTo-Do Items
To add a To-Do List item, open src/components/App.js and place the code below:
class App extends Component {
//add Todo
addTodo = (note) => {
const id = this.state.todos.length + 1;
const newTodo = {
id: id,
note: note,
completed: false
}
this.setState({todos: [...this.state.todos, newTodo]});
}
render() {
return (
<Router>
<div className="App">
<div className="container">
<Header />
<br />
<Route exact path="/" render={props => (
<React.Fragment>
<AddTodo addTodo={this.addTodo} />
<Todos todos={this.state.todos} markComplete = {this.markComplete} delTodo={this.delTodo}/>
</React.Fragment>
)} />
</div>
</div>
</Router>
);
}
}
Step 5 — Deleting and Mark as complete To-Do Items
To delete and mark a To-Do List item as complete, open src/components/App.js and place the code below:
// Mark as Complete Todo
markComplete = (id) => {
this.setState({
todos: this.state.todos.map(todo => {
if(todo.id === id)
todo.completed = !todo.completed;
return todo;
})
});
}
// Delete Todo
delTodo = (id) => {
this.setState({ todos: [...this.state.todos.filter(todo => todo.id !== id)]});
}
Step 6 — Finalizing the App.js
import React, { Component } from 'react';
import { BrowserRouter as Router, Route } from 'react-router-dom';
import Todos from './Todos';
import Header from './layout/Header';
import AddTodo from './AddTodo';
import '../assets/App.css';
class App extends Component {
state = {
todos: [
{
"id": 1,
"note": "Design the solution",
"completed": false
},
{
"id": 2,
"note": "Prepare for implementation",
"completed": false
},
{
"id": 3,
"note": "Prepare the test/QA environment",
"completed": false
},
{
"id": 4,
"note": "Install the product in the test/QA environment",
"completed": false
},
{
"id": 5,
"note": "Prepare the production environment",
"completed": false
},
]
}
componentDidMount() {
}
// Mark as Complete Todo
markComplete = (id) => {
this.setState({
todos: this.state.todos.map(todo => {
if(todo.id === id)
todo.completed = !todo.completed;
return todo;
})
});
}
// Delete Todo
delTodo = (id) => {
this.setState({ todos: [...this.state.todos.filter(todo => todo.id !== id)]});
}
//add Todo
addTodo = (note) => {
const id = this.state.todos.length + 1;
const newTodo = {
id: id,
note: note,
completed: false
}
this.setState({todos: [...this.state.todos, newTodo]});
}
render() {
return (
<Router>
<div className="App">
<div className="container">
<Header />
<br />
<Route exact path="/" render={props => (
<React.Fragment>
<AddTodo addTodo={this.addTodo} />
<Todos todos={this.state.todos} markComplete = {this.markComplete} delTodo={this.delTodo}/>
</React.Fragment>
)} />
</div>
</div>
</Router>
);
}
}
export default App;
The final output will look like this:
In this tutorial, you've created a CRUD To-do list app with ReactJs. Congratulations! You’ve officially built a to-do list app with React.
Here, you will find the full source code: https://github.com/MakdiaHussain/todolist.git
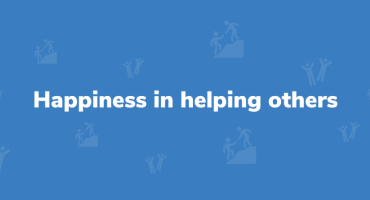
Happiness in helping others
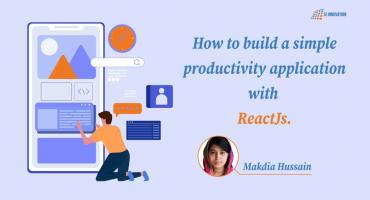
How To Build A Simple Productivity Application With ReactJs
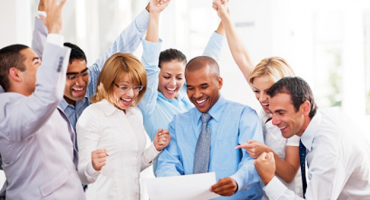