A guide to render a view in AJAX call with Laravel
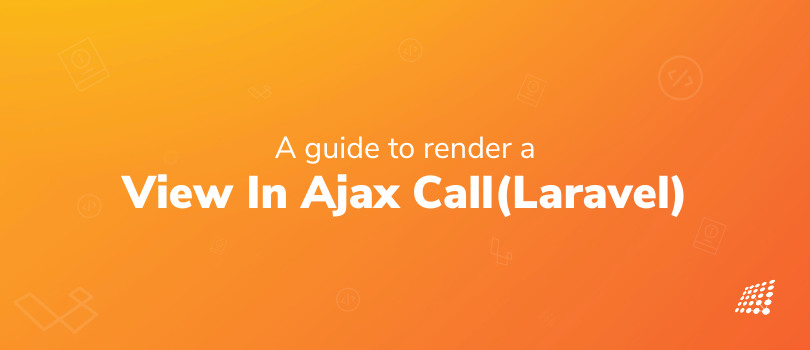
AJAX has revolutionized the way we interact with web applications. After all, look how extensively it is used today for form submissions, dynamic content updates, and data validation tasks. However, have you ever encountered a situation where you wanted to update a portion of your web page without having to refresh the entire page? This is another scenario where the power of Ajax calls comes into play! Yes, it's possible to render views with AJAX and if you are on a quest to take your Laravel development skills to the next level, this guide is for you!
We will be going through the step-by-step process of rendering a view on Ajax's success using Laravel. So whether you're a seasoned developer or just starting out, this guide will show you how to render a view using AJAX with the Laravel framework and provide valuable insights and knowledge on how to make your web applications more dynamic and user-friendly.
Brief AJAX Introduction and Its Uses in Web Development
Let’s first get an introduction to AJAX and an introduction to AJAX in web technology! AJAX, which stands for Asynchronous JavaScript and XML, is the name of a group of web development tools that make it possible to build quick, dynamic, and asynchronous web applications. The user experience is improved and speeded up since it enables developers to update specific sections of a web page without having to reload the full page.
Laravel is a free and open-source PHP web framework for building online applications. It is a popular option among developers owing to its elegant syntax and powerful features. If you’re wondering what is AJAX used for in web pages, you should know that the Laravel framework is a great option for creating AJAX-based web apps because it comes with built-in support for the technology. This blog intends to offer a thorough tutorial on how to render a view in a Laravel AJAX request, assisting developers in utilising the powerful and quick AJAX features that Laravel offers.
Understanding the Basics of an AJAX Call in Laravel
It's crucial to comprehend the basics of an AJAX call in Laravel and understand how they operate within the Laravel framework in order to show a view in an AJAX call in Laravel efficiently. This includes AJAX's fundamental ideas, such as asynchronous requests and dynamic updates, as well as the precise syntax and capabilities made available by Laravel for making AJAX calls.
As a starting point, what can be helpful is learning the fundamentals of an AJAX call in Laravel and how it is applied to web development, as well as the salient characteristics and advantages of the Laravel framework and how they relate to AJAX. After you have this basic understanding, you will be better prepared to use AJAX calls to render views in Laravel for form submissions, dynamic content updates, and other activities.
How to Render a View on AJAX Success (using Laravel)
Now, let’s look at the steps to render a view in AJAX Laravel. We use AJAX to submit forms without refreshing the page. This makes it more interactive to the user. Think of a dynamic dependent select where you select a country depending on the states that you see under that country. This is done by running a select query. In this case we return IDs on AJAX success. However, for rendering views in AJAX Laravel, we can use the "laravel render view ajax" approach. This approach is used to return a view in an AJAX call.
Let’s see how to return a view on AJAX success using the "how to return view in ajax call" approach (using Laravel).
Suppose we have a dropdown select
<form >
<input type="hidden" name="_token" id="token" value="{{ csrf_token() }}">
<label for="job_type">Choose a Job:</label>
<select name="job_type" id="job_type_filter" class="p-2 m-1 rounded text-center">
<option value="1">Intern</option>
<option value="2">QA</option>
<option value="3">HR</option>
<option value="4">Developer</option>
</select>
</form >
Depending on the selected item, it filters out the job and its details in the view .
$(document).ready(function() {
$('#job_type_filter').change(function() {
var jobType = $('#job_type_filter').val();
let requestBody = {
"_token": $('#token').val(),
jobType: jobType,
}
$.ajax({
method: "POST",
url: "/filterjob",
data: requestBody,
datatype: 'json',
success: function(result) {
console.log(result);
$('#filter-out-here').html(result)
}
});
});
});
In the above snippet you see the form is submitted to the filterjob method of the controller. It takes the ID of the job-type when the dropdown is selected.
Controller code:
public function filterByJobType(Request $request)
{
$jobTypeId=$request->jobType;
$jobs = Job::with('position','jobPost','team','location','jobType')->where([['status',false],['job_type_id',$jobTypeId]])->orderBy('id', 'DESC')->get();
}
return view('filterJob',compact('jobs'));
}
In the above code snippet, we see that the controller method receives the job type ID and fetches the data that is required to render in the view, then it returns a view named filterJob.
The View which Displays the Filtered Jobs
<div class="card" style="width: 18rem;">
<h3 class="post-title">Job title - Tech Stack</h3>
<ol class="breadcrumb">
<li><a href="#">Development</a></li>
<li><a href="#">Full Time</a></li>
</ol>
<p class="requirments">Requirements</p>
<ul class="list-group list-group-flush">
<li>- Turn design and user interface mockups into functional websites.</li>
<li>- Work with team, perform requirement analysis and gather requirements.</li>
<li>- Proficient in the maintenance and administration of Drupal modules and sites.</li>
<li>- Custom programming and web application development for Drupal</li>
</ul>
</div>
Traditionally we send json data from a controller then manipulate that data using js. But this makes the rendering is easy because you are just passing the same view while AJAX is a success.
Few Tips for Form Submission in AJAX
Here is what you need to know for form submission in AJAX.
Don’t Forget to Send a CFR Token While Submitting the Form
The CSRF token is a unique, random value that is generated for each form, and it is included as a hidden field in the form.
When the form is submitted, Laravel compares the token included in the submission with the token that was generated for the form.
If the tokens match, the submission is considered to be legitimate, and the request is processed.
If the tokens do not match, the submission is considered to be an attack, and the request is rejected. This helps to prevent unauthorized form submissions on your website.
Don’t Forget to Set the Route in web.php.
If a route is not set for a form submission in Laravel, the form data will not be sent to the server and will not be processed.
Instead, the browser will display an error message indicating that the form's action attribute is missing or that the route does not exist. if you are not defining a route for a form, you will see a 404 error page.
In conclusion to the AJAX overview, this tutorial has shown a step-by-step process to render a view on the success of an AJAX call in Laravel. It opens with a description of AJAX, its importance in web development, and the advantages it has for the Laravel framework.
Understanding the basics of an AJAX call in Laravel, such as its asynchronous requests and dynamic updates, is crucial to make the most of its capabilities.
Following that, we moved on to how you render a view on the success of an AJAX call in Laravel, using a real-world example of filtering jobs based on the chosen job type. By following the steps outlined in this guide, you will be able to enhance the interactivity and user-friendliness of your Laravel web applications successfully.
Ready to take your Laravel web development to the next level? Our experienced and skilled web development experts are here to help! Whether you need guidance on AJAX implementation or require expert assistance with custom web application development, we've got you covered. Contact us today to discuss your project requirements!
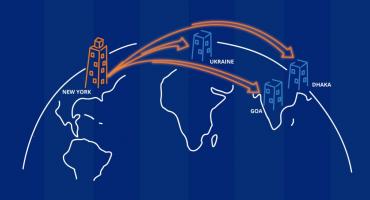
At the Heart of SJ Innovation: How we Coordinate our International venture
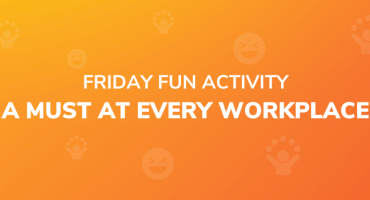
FRIDAY FUN ACTIVITY : A MUST AT EVERY WORKPLACE
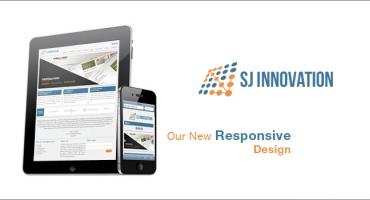