How to create a registration system with email verification using php
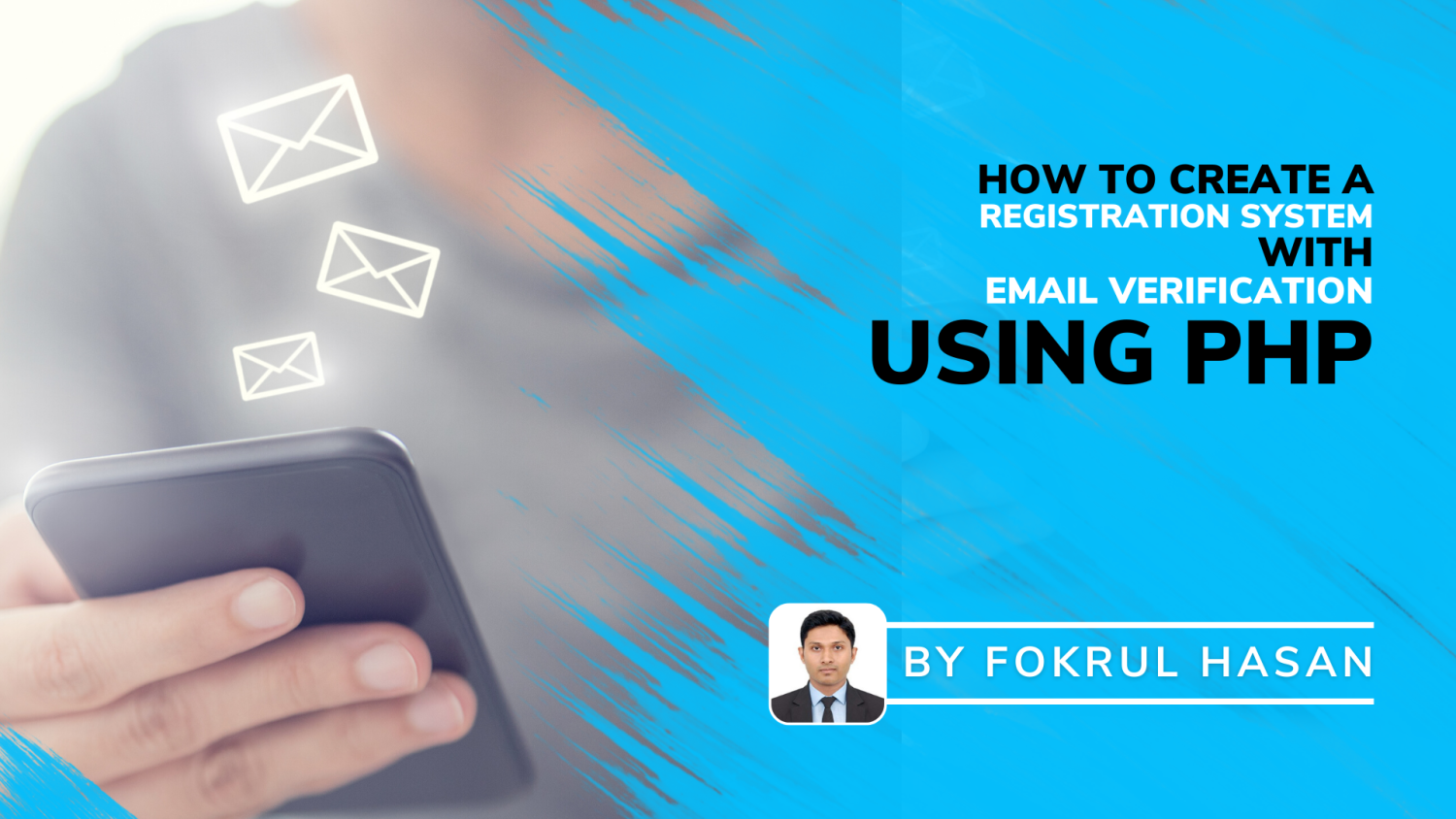
In the digital age, the entry point to any online service is through a seamless registration process. It’s the handshake that begins the relationship between your website and your users. But how do you ensure that this crucial first interaction is both welcoming and secure? Cue PHP, a reliable ally in the web development arena.
Welcome to "how to create an authentication system in PHP?" – your no-nonsense guide that walks you through constructing a user registration pathway that’s not just user-friendly but also armored against security breaches.
Step-by-Step Guide on How to Create an Authentication System in PHP
In this article, I will tackle the essential task of how to verify email with a verification code using PHP, while also discussing how to send an email from localhost. Additionally, we'll delve into crafting a secure registration system that incorporates email verification using PHP to safeguard your user's digital interactions.
In creating our PHP registration system, I will focus on using procedural or 'row' PHP, steering away from object-oriented programming (OOP). For efficiency and a touch of style, the user registration PHP script will be enhanced with Bootstrap code to expedite development and ensure responsiveness.
Additional things we will need:
- phpMailer library
- mailtrap.io account credential for sending emails
First let’s create a database and a table. Database name is `verification` and table name is `users`.
The columns which I will create to validate is,
Id, email, password,code, is_verified.
This is the code to create the table.
CREATE TABLE `verification`.`verification` ( `id` INT(11) NOT NULL AUTO_INCREMENT , `name` VARCHAR(50) NOT NULL , `email` VARCHAR(60) NOT NULL , `code` VARCHAR(10) NOT NULL , `is_verified` TINYINT(3) NOT NULL DEFAULT '0' , PRIMARY KEY (`id`)) ENGINE = InnoDB;
Now let's move to the coding part.
First I am creating a folder named as verification inside my local server folder. It might be www/htdocs based on your server app(wamp/xampp/ampps etc )
Now inside this `verification ` folder I am creating those files.
- Registration.php
- Verify.php
- Db.php
- sendEmail.php
Let's initiate our journey by setting up 'db.php.' In this foundational file, we'll establish a connection to the database necessary for our email confirmation script. This crucial step paves the way for further PHP user authentication processes in our system.
Below, you'll find the pertinent code for 'db.php'
<?php
$servername = "localhost";
$username = "root"; // user name for the server
$password = "mysql"; // password for the server
$conn = mysqli_connect($servername, $username, $password);
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
?>
So our database is connected now.
With our database now connected, we'll advance our secure registration PHP process by integrating email functionality. To to download it go to this link: PHPMailer/PHPMailer: The classic email sending library for PHP (github.com)
Once downloaded, rename it to PHPMailer and place this folder within our project directory. Our project, aptly named verification, will now house the PHPMailer, reinforcing our secure PHP registration system with email confirmation capabilities. Place the PHPMailer folder inside the verification directory to proceed.
So our project folder looks like this.
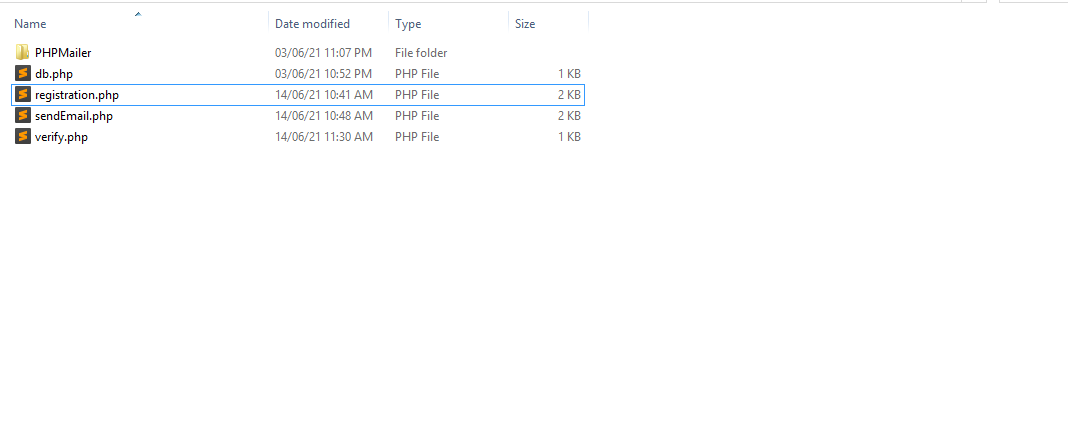
Now our mailer library is also integrated. Let’s configure the mailer code.
Before configuring, let's get the mailtrap.io credential. To get this, login to your mailtrap.io account and get the credential as mentioned in the screenshot below.
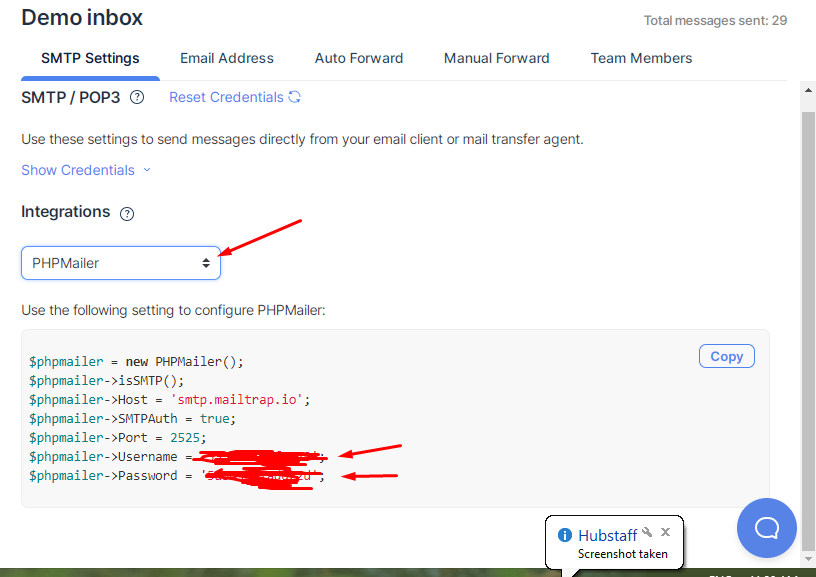
Now open sendEmail.php and paste the below code.
<?php
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
class sendEmail
{
function send($code)
{
require 'PHPMailer/src/Exception.php';
require 'PHPMailer/src/PHPMailer.php';
require 'PHPMailer/src/SMTP.php';
// create object of PHPMailer class with boolean parameter which sets/unsets exception.
$mail = new PHPMailer(true);
try {
$mail->isSMTP(); // using SMTP protocol
$mail->Host = 'smtp.mailtrap.io'; // SMTP host as gmail
$mail->SMTPAuth = true; // enable smtp authentication
$mail->Username = ''; // sender gmail host
$mail->Password = ''; // sender gmail host password
$mail->SMTPSecure = 'tls'; // for encrypted connection
$mail->Port = 587; // port for SMTP
$mail->isHTML(true);
$mail->setFrom('[email protected]', "Sender"); // sender's email and name
$mail->addAddress('[email protected]', "Receiver"); // receiver's email and name
$headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n";
$mail->Subject = 'Email verification';
$mail->Body = 'Please click this button to verify your account: <a href=http://localhost/verification/verify.php?code='.$code.'>Verify</a>' ;
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) { // handle error.
echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo;
}
}
}
$sendMl = new sendEmail();
?>
In the above code, we will need to update our mailtrap.io username and password. So using the above screenshot, add your mailtrap.io credential.
Those are the two lines which we need to update with our own mailtrap account
$mail->Username = ‘’;
$mail->Password = ‘’;
So our email sending function is ready.
Now we will work on registration.php. I won’t do any email or password validation here.
Copy the below codes and paste inside registration.php file
<?php
include('db.php');
include('sendEmail.php');
if (isset($_POST['submit'])) {
$email = $_POST['email'];
$password = $_POST['password'];
$code = rand();
$sql = "INSERT INTO `verification`.`users` (`id`, `password`, `email`, `code`) VALUES (NULL, '$password', '$email', '$code')";
$result = mysqli_query($connection,$sql);
if ($result) {
echo "Registration successfull. Please verify your email.";
$sendMl->send($code);
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<h2>Reg form</h2>
<form action="" method="post">
<div class="form-group">
<label for="email">Email:</label>
<input type="email" class="form-control" id="email" placeholder="Enter email" name="email">
</div>
<div class="form-group">
<label for="pwd">Password:</label>
<input type="password" class="form-control" id="pwd" placeholder="Enter password" name="password">
</div>
<button type="submit" name="submit" class="btn btn-primary">Submit</button>
</form>
</div>
</body>
</html>
Now run your registration.php file. I will run it like this.
`localhost/verification/registration.php `
It should show a form like this:
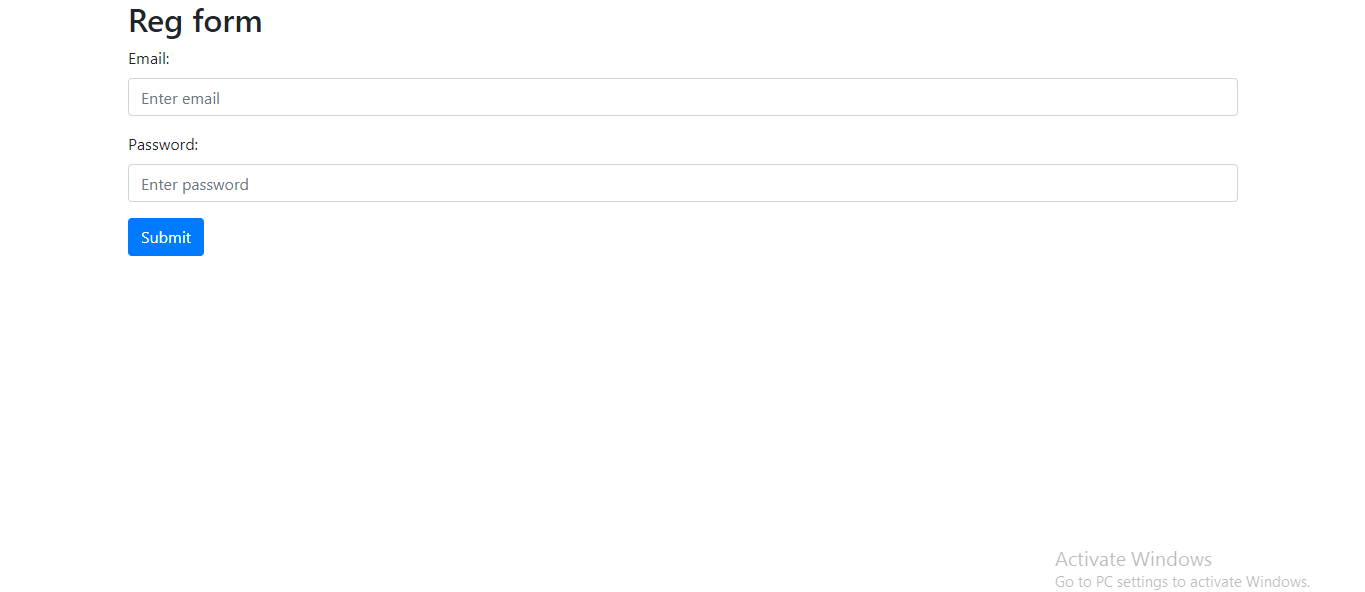
Now fill the form and hit submit. If everything you did was fine, then it should save the data into the database. Also you should see an email that got sent to your mailtrap.io account as shown below.
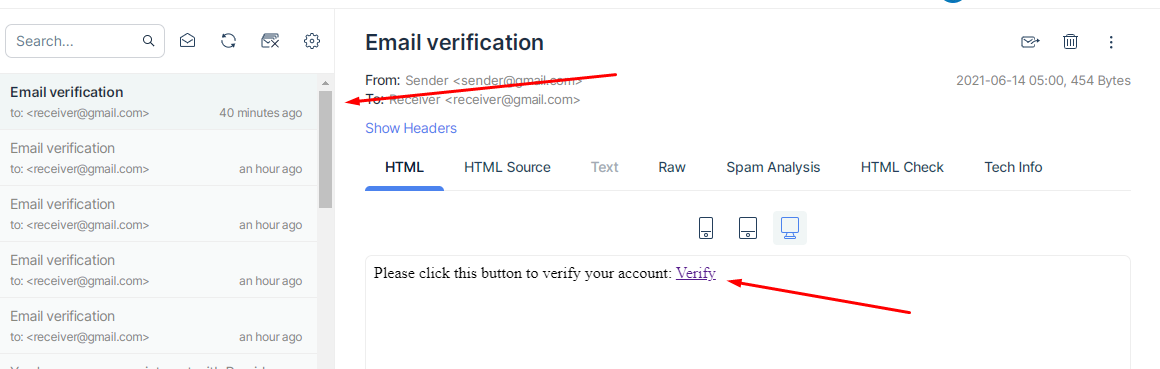
So our registration is done.
Now we will be working on verification.php
Paste the below code inside verify.php
<?php
include('db.php');
if (isset($_GET['code'])) {
$id = $_GET['code'];
$sql = "UPDATE `verification`.`users` SET is_verified='1' WHERE code =$id";
$result = mysqli_query($connection,$sql);
if ($result) {
echo "Your account is verified";
}
}
else {
$message = "Wrong url";
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<?php
echo $message;
?>
</div>
</body>
</html>
Now click on the link sent to your mailtrap account and your account will be verified.
Please note, that I had skipped validation and some standard checking such as password matching because the main intention was to send the email from local and make a registration system where email verification is required.
As we conclude this guide on implementing email verification in PHP user authentication, it's critical to recognize the significance of establishing secure digital interactions. Throughout this blog, we've navigated the complexities of PHP scripts for signup forms with email verification, integrating the PHPMailer library, and harnessing mailtrap.io to simulate flawless email delivery.
You can also watch our latest video on How to Test PHP Code With PHPUnit
By demystifying these processes, our goal has been to enable you not only to grasp the concepts behind a secure registration system but also to apply them confidently to your projects. Your digital creations deserve to be as trustworthy as they are innovative. As you continue to build and enhance your web presence, remember that a reliable and secure PHP framework is the cornerstone of a thriving online service.
FAQs
1. How to Create an Authentication System in PHP?
Set up your database: Create a users table in your MySQL database with fields for storing usernames, passwords (hashed), and any other necessary information.
Connect to the database: Utilize PHP's PDO (PHP Data Objects) or mysqli to connect to your MySQL database from your PHP script.
Create registration and login forms: These will be used for users to input their credentials.
Handle the form data: Write PHP scripts to process the submitted data, checking against the database for matches.
Password handling: Store passwords securely using password hashing functions like password_hash() and verify them with password_verify().
Session management: Use PHP sessions to keep track of logged-in users.
Remember to use secure practices such as prepared statements to prevent SQL injection and HTTPS to encrypt the data transmission.
2. How to Fetch Data from the Database in PHP for a Login Page?
To fetch user data for login purposes:
Establish a database connection: Use mysqli_connect() or create a PDO instance to connect to your MySQL database.
Prepare a SQL query: Write a SELECT statement querying the users table by username or email. Use prepared statements to prevent SQL injection.
Execute the query: With PDO or mysqli, execute the query and fetch the results.
Verify user input: Check if the input password matches the hashed password stored in the database with password_verify().
Initialize session: Upon successful login, initiate a session for the user.
Always ensure your connection uses PDO or mysqli with prepared statements for security.
3. How to Use Session in PHP for Login and Logout?
For Login
Start the Session: Begin your login script with session_start();. This tells PHP to start tracking the session.
Store User Info: After the user logs in successfully, save their information in the session so it keeps the user logged in as they move around the site.
For Checking Login Status on Other Pages: Start the Session: At the start of each page, use session_start(); again.
Check If Logged In: Before showing the page, check if the user is logged in.
If they're not, send them back to the login page.
For Logout
Clear the Session: To log out, clear the user's session. Redirect: Send the user back to the login page or the homepage after they've been logged out.
Dive into our easy-to-follow PHP guide now and transform your registration system with robust email verification. Don't just learn—implement! Click here to begin your journey to a safer digital world. Your code. Your rules. Secure it today!
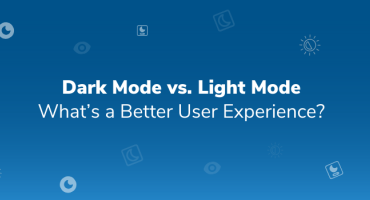
Dark Mode vs. Light Mode: What’s a Better User Experience?
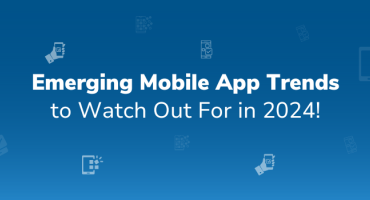
Emerging Mobile App Trends to Watch Out For in 2024!
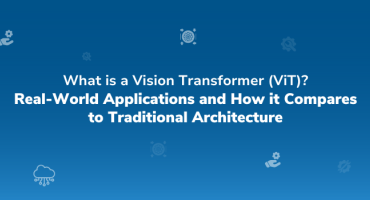