How Error & Exception Handling Works in PHP Framework with Examples
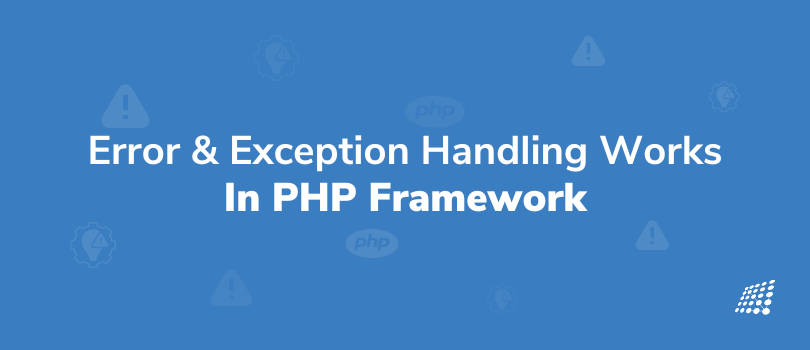
Error and exception handling in PHP are powerful mechanisms. While exception handling helps change the usual flow of the code execution if a specified error occurs, procedural error handling in PHP detects errors raised by your program and then takes the necessary course of action. How does this help though?
In this article, we discuss error & exception handling and throw light on why it matters. In the first half of the article, we talk about error handling in PHP and how it works. In the second half, we show you exception handling by giving real-time examples.
What is Error Handling?
Error handling is a way of catching errors generated by your program/code and then taking appropriate steps to solve this error. If you handle errors properly then your program will run smoothly without any failures and system crashes. It's very easy in PHP to handle errors.
A PHP error occurs when something is wrong in your PHP code. For example, it can be a missing semicolon, calling an incorrect variable or file does not exist, etc.
There are four types of errors in PHP when dealing with PHP error handling best practices and they are as follows:
1. Warning Error
2. Notice Error
3. Parse Error
4. Fatal Error
Now I will give you an idea of all these errors with examples:
1. Warning Error:
A warning error is a type of error that does not stop the execution of the script, it only warns you that there is a problem in your program which might cause bigger issues in the future.
The main reason for warning errors is to include a missing file or passing an incorrect number of parameters in a function.
Example:
<?php
echo "Warning Error!!";
include ("function.php");
?>
Output
In the above code, we include a function.php file but the function.php file does not exist in the project directory. So, in that case, there will be a warning error produced but it does not stop the execution of the script i.e. you will see a message for "Warning Error !!" and after the warning, you will see another message for "Below the Warning Error Section!!".
Like the following image:
2. Notice Error
Notice error and warning error both are similar which means it does not stop the execution of the code.
It occurs when you try to access an undefined variable, then it generates a notice error.
Example
<?php
$a=10;
echo "Notice Error !!";
echo $b;
echo "Below notice error";
?>
Output
In the above code, we defined a variable named $a. But we call another variable $b, which is not defined. So it will produce a notice error but it does not stop the execution of the script i.e. you will see a message "Notice: Undefined variable: b" and after this notice error you will see another message for "Below notice error!!". Like the following image:
3. Parse Error
A Parse error is a type of error that you need to know about when dealing with PHP error handling best practices and is caused by misused or missing symbols in a syntax. A parse error is also known as a Syntax error. The compiler catches the error and stops/terminates the execution of the script. After fixing the syntax error the compiler compiles the code and executes it.
Some common reasons for parse errors are:
- Unclosed quotes/braces
- Missing or Extra parentheses/semicolon
- Misspellings
Example
<?php
echo "A";
echo "B"
echo "C";
?>
Output
In the above code, we missed the semicolon in the second line. As a result, when the compiler compiles the code it will produce a parse or syntax error which stops the execution of the script i.e. you will see a message "Parse error: syntax error". Like the following image:
4. Fatal Error
A fatal error is another type of error that is caused due to the use of an undefined function or class. That means if you are trying to access an undefined function or class, then it will generate a fatal error and it will stop the execution of the script.
It is the type of error where the PHP compiler understands the PHP code but it recognizes an undeclared function. This means that function is called without the definition of the function.
Example
<?php
function message()
{
echo "Hello team";
}
sms();
echo "Fatal Error !!";
?>
Output
In the above code, we defined a function named message but we call another function sms which is not defined. So it will produce a fatal error that stops the execution of the script. Like the following image:
Ways to Handle PHP Errors
Procedural error handling in PHP can be handled in the following ways:
- Using die() method
- Custom Error Handling
1. Using die() function
I am writing a script that opens a text file. If the file does not exist then it might produce an error like the below example:
<?php
$file=fopen("profile.txt","r");
?>
Now to prevent the user from getting the error message like the above one, first, we will check whether the file exists or not before accessing it. Now if the file does not exist you will get a message like the below image:
<?php
if(!file_exists("profile.txt")) {
die("File not found");
}else {
$file = fopen("profile.txt","r");
print "Open file successfully";
}
?>
So the code above is more efficient than the previous code because it uses a simple error handling mechanism to stop the script after the error.
2. Custom Error handling
Custom error handling in PHP is quite simple. you can create a function that can be called when an error has occurred in PHP.
Function syntax
error_function(error_level,error_message,error_file,error_line,error_context);
The above function must be able to handle a minimum of two parameters (error level and error message) but can accept up to five parameters (optionally: file, line-number, and the error context):
Parameter & Description
$error_level: Required parameter and it must be an integer. There are predefined error levels.
$error_message: Required parameter and it is the message which the user wants to print.
$error_file: Optional parameter and used to specify the file in which error has occurred.
$error_line: Optional parameter and used to specify the line number in which error has occurred.
$error_context: Optional parameter and used to specify an array containing every variable and their value when an error has occurred.
Example
<?php
function myerror($error_no, $error_msg) {
echo "Error: [$error_no] $error_msg ";
echo "\n";
echo "Now Script will end";
die();
}
set_error_handler("myerror"); // Setting set_error_handler
$a = 50;
$b = 0;
echo($a / $b); // This will generate error
?>
Output
In the above code, we tried to divide 50 by 0, so it will produce an error. So here we tried to handle the errors using custom error handling as it will show more specified messages. If we don't handle this using Custom error handling then it will generate an error and stop the script execution but if we handle the error using Custom error handling so it can continue the script after displaying the error message.
Exceptions Handling
In PHP, exception handling is a powerful way which can handle runtime errors. So runtime errors are also known as exceptions. It's a signal which indicates some errors may occur due to various reasons such as database connection failing, the file doesn't exist, etc. So the main purpose of using exception handling is to maintain the normal execution of the application. As an exception interrupts the normal flow of the application. But it is different from an error because an exception can be handled, whereas an error cannot be handled by the program itself.
In other words, - "An unexpected result of a program is an exception, which can be handled by the program itself." Exceptions can be thrown and caught in PHP.
Benefits of Exception Handling
- Exception handling can control run-time errors that occur in the program.
- It can avoid abnormal termination of the program and also shows the behavior of the program to users.
- It can separate the error handling code and normal code by using try-catch block.
PHP offers the following keywords for handling exceptions:
Try
The try block contains the code that may have an exception or where an exception can occur. When an exception occurs inside the try block during the runtime of the program, it is caught and resolved in the catch block. The try block must be followed by a catch or finally block. A try block can be followed by nested catch blocks.
Catch
The catch block contains the code that executes when a particular exception is thrown. It is always used with a try block, not alone. When an exception occurs, PHP finds the matching catch block.
Throw
It is a keyword that is used to throw an exception. It also helps to list all the exceptions that a function throws but does not handle itself. Remember that each throw must have at least one "catch".
Finally
The final block contains a code, which is used for clean-up activity in PHP. It executes the essential code of the program.
Example of exception handling in PHP
One example of exception handling is Divide By Zero. Here, we will use try, catch, and throw keywords to demonstrate the DivideByZero exception in a PHP program.
Example
<?php
try
{
$firstValue = 10;
$secondValue = 0;
if ($secondValue == 0) throw new Exception("Divide by Zero exception occurred");
$result = $firstValue / $secondValue;
printf("Result is : ", $result);
}
catch(Exception $e)
{
printf("Exception: %s", $e->getMessage());
}
?>
Output
Exception: Divide by Zero exception occurred
The above code demonstrates the Divide By Zero exception using exception handling. Here, we used try and catch blocks. In the try block, we created two local variables $firstValue, $secondValue that are initialized with 10 and 0 respectively.
if($secondValue==0)
throw new Exception("Divide by Zero exception occurred");
Here we check the condition, if the value of $secondValue is 0 then it will throw an exception using the throw keyword that will be caught by the catch block and print specified message. Also, the program will be compiled and executed successfully.
A Real-World Example
In this section, we'll know about a real-world example to demonstrate exception handling in PHP.
Let's think you've built an application that loads the application configuration from the config.php file. Now, the config.php file must be present when your application is bootstrapped. Thus, your application can't run if the config.php file is not present. So this is the perfect example to throw an exception and let the user know they need to fix the issue.
<?php
try {
// init bootstrapping phase
$configFilePath = "config.php";
if (!fileExists($configFilePath))
{
throw new Exception("Configuration file not found.");
}
// continue execution of the bootstrapping phase
} catch (Exception $e) {
echo $e->getMessage();
die();
}
?>
As you can see in the above example, we're checking for the existence of the config.php file at the beginning of the bootstrapping phase. If the config.php file is found, the execution continues normally. On the other hand, we'll throw an exception if the config.php file doesn't exist. Also, we would like to stop execution if there's an exception!
We hope these error & exception handling in PHP framework example instances have helped you master what you need to do when dealing with these aspects fruitfully! For more information, get in touch with our experts at SJ Innovation.
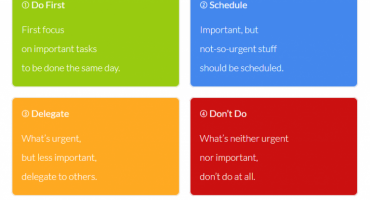
Be productive using tools and techniques.
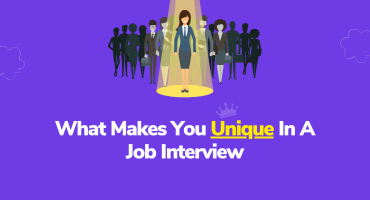
What Makes You Unique In A Job Interview
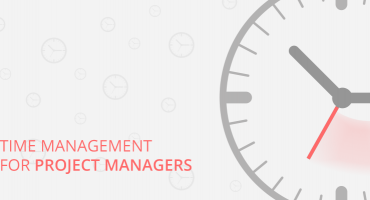