Mastering the Art of Hooks: A Guide to Enhancing Functional Components In React
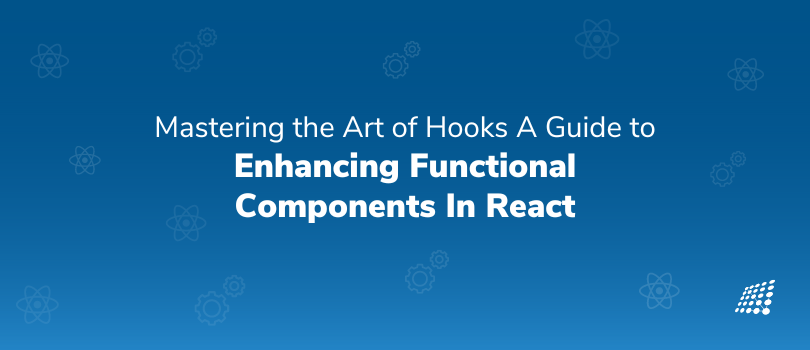
Are you tired of your functional components falling flat and failing to engage your users? Well, in this comprehensive guide, we’ll talk about how to master the art of hooks and react hooks benefits, taking your functional components to the next level.
React functional components are a powerful tool for building user interfaces in React. They allow you to write functional, reusable, and composable components that can be easily integrated into your application. In this blog post, we will discuss the use of hooks in functional components, and how they can be used to manage state and side effects in your application.
What are Hooks?
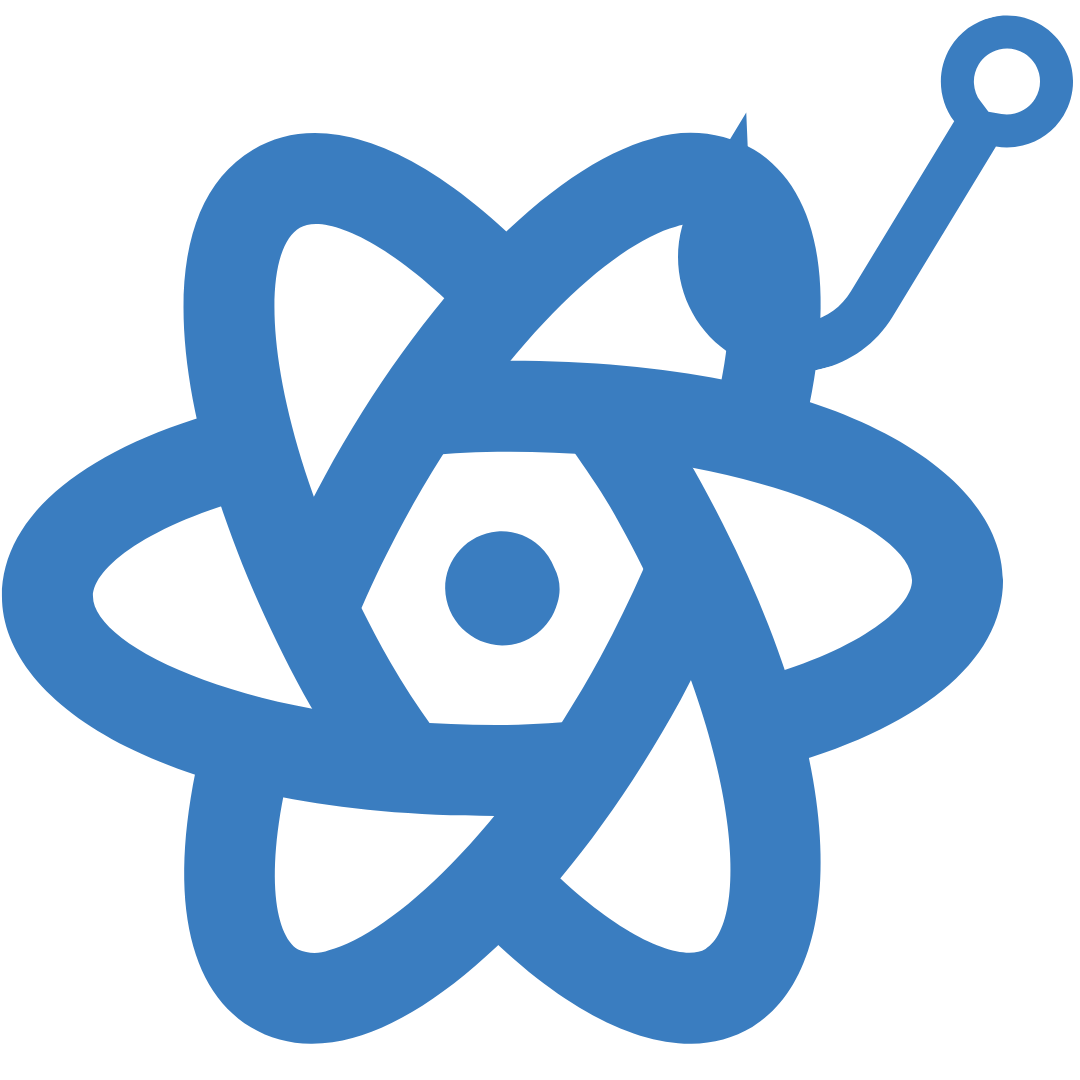
What are the hooks in React? Hooks are a new and powerful feature in React that allows you to use state and other React features in functional components without writing a class. Prior to hooks, only class components were able to use state and lifecycle methods. React Hooks benefits a lot! For instance, with hooks, you can now use state and other features in functional components, making them more versatile and easy to use. By understanding and utilizing hooks effectively, you can enhance the functionality and interactivity of your components, resulting in a more engaging user experience.
The Upside of Using Hooks
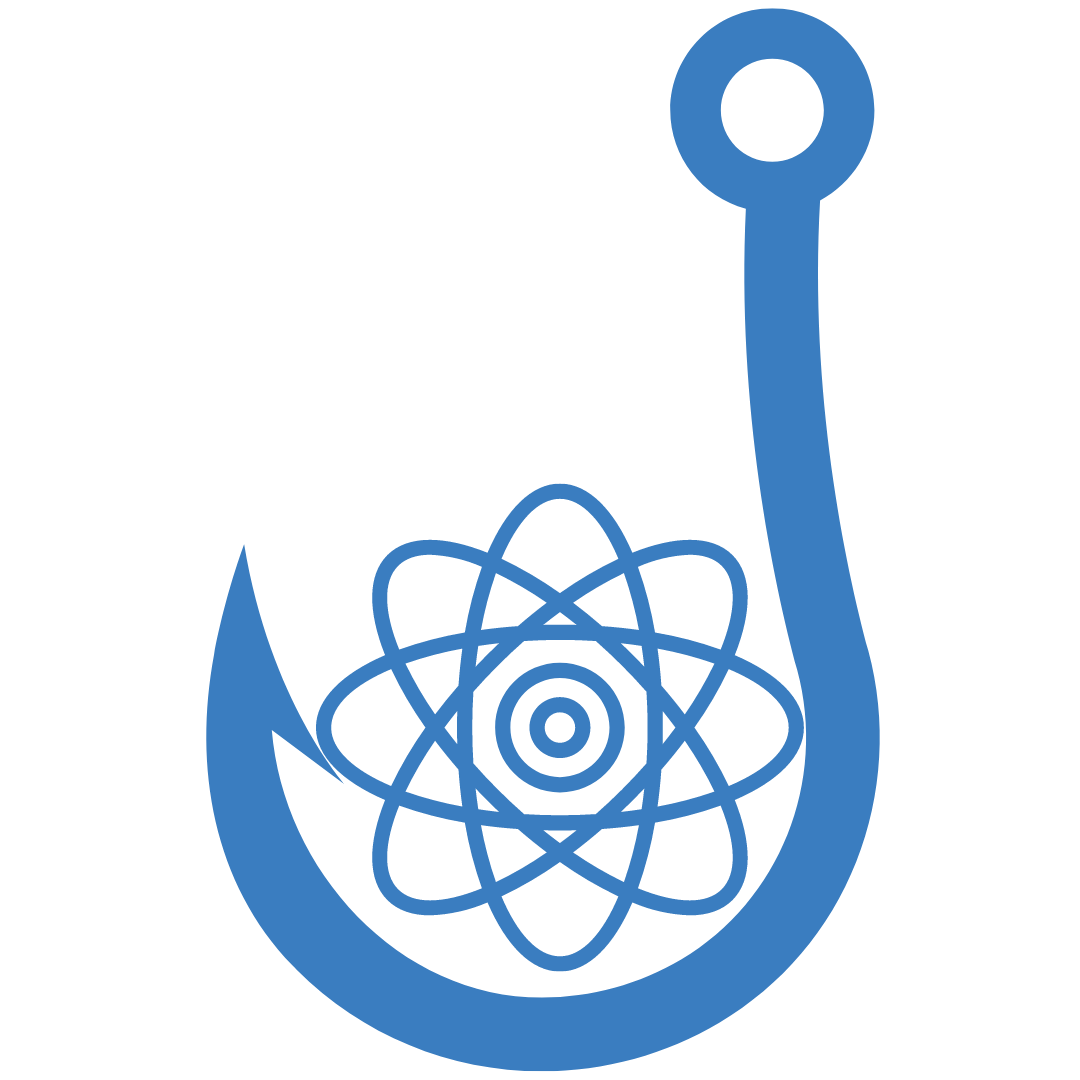
Streamlined Development
- There are many react functional components best practices. Hooks bring a breath of fresh air to functional components, enabling a more streamlined and intuitive development experience.
- The use of hooks in functional components make it easy to write clean, concise code that is easier to read, understand, and maintain.
- Wondering about the use a hook in a class component? Well, say goodbye to the verbosity of class components and embrace the simplicity and elegance of hooks.
Reusability and Composition
- Hooks empower you to create reusable and composable code.
- Another among the react hooks benefits is that you can build custom hooks that encapsulate specific functionality, allowing you to reuse them across multiple components.
- This modular approach fosters code reuse, reduces duplication, and promotes a cleaner architecture.
- With hooks, you can compose complex functionality by combining smaller, focused hooks, resulting in a more maintainable and scalable codebase.
Enhanced Code Organization
- One of the main React hooks benefits is that it provides a natural and intuitive way to organize your code.
- Instead of spreading logic across different lifecycle methods or higher-order components, hooks allow you to group related code together in a single function.
- This improves code readability and makes it easier to locate and modify specific functionality within a component.
Simplified State Management
- Managing state in functional components becomes a breeze with hooks.
- The useState hook grants you the power to effortlessly handle component-level state, eliminating the need for class components and constructor functions.
- By using hooks, you can declare state variables and update them with ease, enhancing the reactivity and responsiveness of your components.
Handling Side Effects
- Hooks, such as useEffect, provide a straightforward mechanism for handling side effects in functional components.
- Whether it's making HTTP requests, subscribing to event listeners, or manipulating the DOM, hooks allow you to encapsulate side-effect logic in a clean and predictable way.
- This improves code readability and maintainability, making it easier to reason about the behavior of your components.
Seamless Migration
- If you're already working with class components, fear not, as hooks offer a seamless migration path.
- Among the React hooks benefits is that you can gradually introduce hooks into your existing codebase without the need for a complete rewrite.
- This flexibility allows you to embrace the power of hooks at your own pace, making the transition smoother and more manageable.
Vibrant Community and Ecosystem
- Since hooks were introduced, they have gained immense popularity within the React community.
- This popularity has fostered a vibrant ecosystem of hooks, libraries, and resources.
- You can find a plethora of hooks that cater to specific use cases, saving you time and effort when implementing common functionalities.
- Additionally, the supportive community provides valuable insights, best practices, and troubleshooting assistance, ensuring you're never alone on your hook-infused journey.
How to use Hooks in Functional Components
Wondering how to use hooks in functional components? First, you first need to import the useState and useEffect hooks from the react library. The useState hook allows you to add state to your functional component, while the useEffect hook allows you to handle side effects, such as fetching data or updating the DOM.
Let’s look at how to use hook in React. More specifically, here is an example of a functional component that uses the useState hook to manage a local state variable:
Copy code
import { useState } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
In this example, the useState hook is used to create a local state variable called count, which starts at 0. The setCount function is used to update the count state variable when the button is clicked.
The useEffect hook can be used to handle side effects in functional components. This can be useful for fetching data from an API or updating the DOM when a component is rendered.
Here is an example of a functional component that uses the useEffect hook to fetch data from an API:
Copy code
import { useEffect, useState } from 'react';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://my-api.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
return (
<div>
{data && <p>{data.message}</p>}
</div>
);
}
In this example, the useEffect hook is used to fetch data from an API when the component is rendered. The useEffect hook takes a function that is called when the component is rendered, and an array of dependencies that are used to determine when the effect should be re-run. In this case, the effect is only run once, when the component is first rendered because the dependencies array is empty.
Hooks are a powerful feature in React that allows you to use state and other features in functional components. They make functional components more versatile and easy to use, and can be used to manage state and side effects in your application. With hooks, you can write functional, reusable, and composable components that can be easily integrated into your application. It's important to keep in mind that hooks need to be used in the exact order as they are declared, and it's not allowed to use hooks inside loops, conditions, or nested functions. If you need to know more about React development or are looking for ways how to use React functional components effectively, get in touch with our web development company in New York
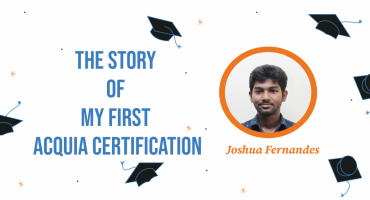
The Story of My First Acquia Certification
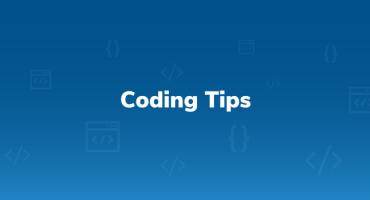
Coding Tips, Duke Experience
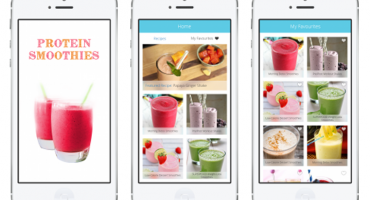