Session And Security in PHP
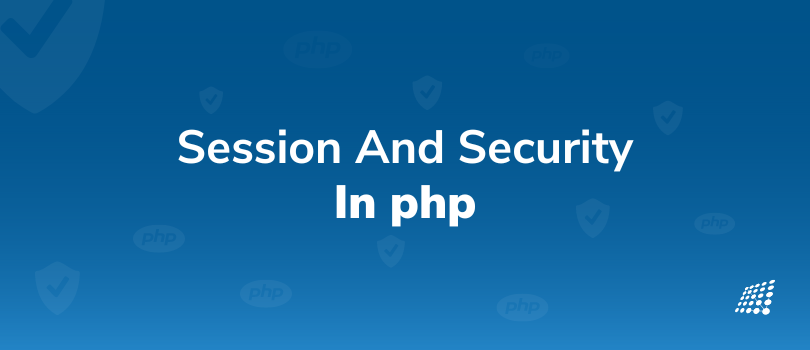
Introduction
On average within the US, internet users access over 130 websites per day. But have you ever thought about how the web server identifies you? This is down to the session variable that stores user information across multiple pages, making your browsing experience seamless.
Getting to understand session and security in PHP MySQL and session management using a database can be confusing. This blog covers the basics of sessions, cookies, and hijack prevention with clear coding examples to guide you through the process.
Session
A session is a global variable stored on the server and used to identify a particular user in that session. One session and security in PHP example is Facebook. Once you log into Facebook, personalized displays are shown based on your previous sessions.
Session vs cookies
Cookies are stored on the local machine of the user. Whereas sessions are saved on the server. Cookies are independent whereas sessions are dependent on cookies. The session needs to be destroyed and cookies when created are given an expiry time.
Session Life cycle:
A PHP session authentication undergoes stages like start, read, write, unset, and destroy. The server creates a unique number for every new session. In order to get your session ID, you can use the session_id function.
<?php
session_start();
echo session_id();
?>
Also you can preset a session by passing an argument to the function in order to create a custom session id.
<?php
session_id(YOUR_SESSION_ID);
session_start();
?>
<?php
// start a session
session_start();
// initialize a session variable
$_SESSION['user_id'] = '171';
// unset a session variable
unset($_SESSION['user_id']);
//destroy the current session
session_destroy();
?>
The unset function is used if we want to delete specific session variables.
Whereas destroy is used to delete all data within the current session.
But in order to delete all global variables associated with the session variable, you must unset the session first.
When you log in to an application it generates a session ID (PHP) and then for logging out, your session id is unset and destroyed.
Regenerate Session Id After You Log In
<?php
session_start();
$old_sessionid = session_id();
session_regenerate_id();
$new_sessionid = session_id();
echo "Old Session: $old_sessionid<br />";
echo "New Session: $new_sessionid<br />";
?>
This way you keep user session information and also regenerate session ID.
Session Hijacking Attack And what Is done with the Hijacked Session
Session Hijacking happens when a malicious party gains access to any user’s session. The attacker will first get the session ID belonging to another user. Then he will use this ID to gain full access to the other user’s session and get authorized to do specific actions under the session ID. Session Hijacking is done by prediction or brute forcing.
Once an attacker gains access to the session that belongs to a particular user, the server will grant him all authority that the original user has.
Measures you may take to secure your session ID
Session ID length
Session identifiers should be at least 128 bits long to prevent brute-force session
Session time out (Session error in PHP)
For low-risk apps, we can keep a timeout for 2 to 5 minutes.
<?php
//session gets destroyed after 2 minutes
if (isset($_SESSION['start']) && (time() - $_SESSION['start'] > 120)) {
session_unset();
session_destroy();
echo "session is destroyed after two minutes";
}
?>
In the above snippet, we destroyed the session after 2 minutes.
Other ways to secure session variables are described above while describing vulnerability.
Types of Session Hijacking Attacks And Measures to prevent
Session Fixation– happens when applications don't insist on setting up a new session ID during authentication. In this type of attack, the attacker forges a valid, but unused Session ID, and then, through various ways, make a valid user authenticate using that ID. To defend against session fixation, we are to assign a different session cookie immediately after a user authenticates to the web, and also verify they do not include the cookie value in the URL.
XSS or Cross-Site Scripting – this attack happens when a third party injects javascript into the body of a website, which is visited by the victim.
In order to prevent that that any user input, through forms or GET parameters, needs to be sanitized before it is used.
In PHP there are two built in primary functions, htmlspecialchars() and strip_tags(), to protect from cross-site scripting attacks.
Session Sidejacking – this attack happens with Man In The Middle attack approach – in situations when the attacker is positioned between the source and the destination of the traffic. The attacker will listen to requests and responses, and access Session ID when it is not encrypted.
In order to prevent it, SSL can be installed to make the whole request encrypted.
Session prediction - this type of attack usually predicts how a session ID is generated and replicates the process to generate a session ID. In order to defend we need to bring uniqueness in generating session ID.
Detecting whether or not generated session ID is Secured
Check whether or not your session id is secured. If any of the following is yes, you are prone to any session vulnerability described above.
- the session ID is not unique or not.
- If the session ID is set from a user-controlled input or not.
- If the session ID is generated with a secure process or using a random generator.
- If the session ID length is too short or not.
Handling session is important as our website get exposed to visitors and attackers. We need to take these described measures in order to make our website free from session hijacking attacks.
To conclude, by adopting several PHP session management best practices, you can actively work against hijacking attacks and secure your session ID. Often learning how to change session id after login in PHP can further aid in reducing session prediction. The PHP session cookie secures the session to provide an additional defense against hijacking attempts.
Want to ensure the security of your PHP sessions? Get in touch with our experts to show you the measures you can take to secure your session ID, and more!
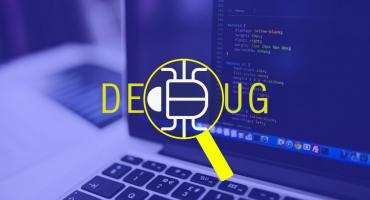
Why Do We Debug Code?
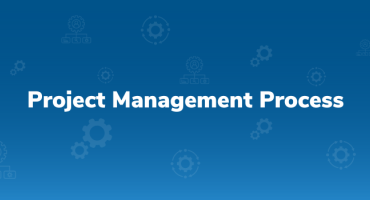
Sjinnovation’s Project Management Process
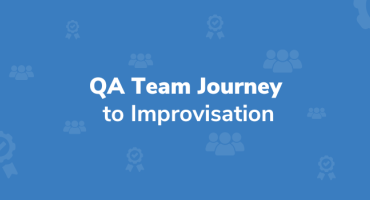