Add and Remove Form fields dynamically with React and React Hooks
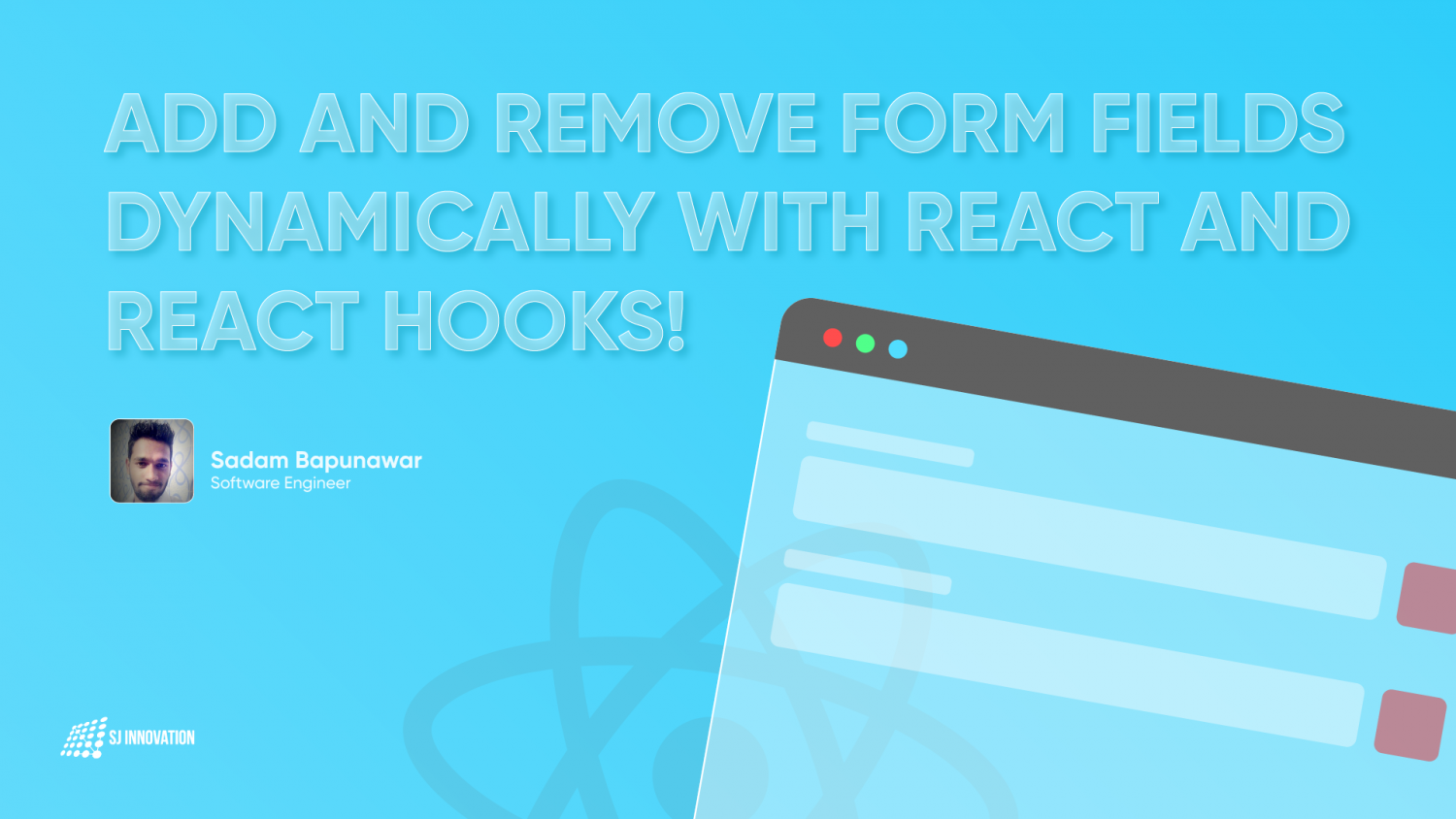
In this guideline, we'll learn:
- Why is it Useful to Dynamically Add and Remove Form Fields
- How to Dynamically Add and Remove Form Fields using React and React Hooks
Web forms are an essential component of any modern website or application, and with the power of React in Laravel 9, completing them can be made even easier. Let's face it, though, completing forms may be time-consuming and annoying, especially if they are lengthy and rigid. For this reason, it's vital to make the process of filling out forms simple, intuitive, and feasible. And what better way to accomplish that than by enabling users to dynamically add and remove form fields?
'In this tutorial, we'll learn how to use React and React Hooks to accomplish this feat. We'll concentrate on React functional components because they are small, effective, and simple to utilize. If you're wondering about the best way to create dynamic forms and the pros and cons of Laravel vs React, we'll guide you through the process of making an informed decision. You can select the strategy that works best for you because we will also supply the entire code to implement this logic using class components. So without further ado, let's go for it!
If you want to know what is React & React Hooks, check this video:
Why is it Useful to Dynamically Add and Remove Form Fields?
Before looking at how to add and remove form fields dynamically with React and React Hooks, let’s look at why it helps to do it and when it might be necessary, especially when comparing popular frameworks like Laravel vs React.
When Collecting user input
When it comes to implementing dynamic form fields, developers may choose to use different tools and frameworks depending on their project requirements. For instance, if you are building a PHP-based web application, you may opt to use Laravel to handle the backend logic, while using React to manage the frontend components.
- Dynamically adding and removing form fields might be helpful when you need to gather user input that may vary in quantity or type.
- For instance, you may need to compile a list of objects that the user can modify as necessary. Or, depending on the demands of the user, you may need to gather a variety of addresses, phone numbers, or other pieces of information.
So rather than looking at Laravel vs React, you can leverage the power of Laravel and React together to design robust web applications that are flexible and easy to use. You can develop scalable and secure backends using Laravel, and you can construct dynamic, responsive user interfaces with React in Laravel 9. You can build web applications that cater to the needs of your users and offer a seamless user experience by having the option to add and remove form fields dynamically.
To Make the Form user-friendly and Reduce Errors
Whether Laravel vs React framework, you can make the form more user-friendly and versatile while also streamlining the user input process by enabling users to dynamically add or remove form fields. This technique can be particularly useful when working with complex data structures, such as arrays or objects, and can be applied to web development frameworks such as React in Laravel 9. Users can add or remove fields as necessary to effectively represent data, which can improve the user experience overall and possibly reduce mistakes or wrong entries.
When Dealing With Complex Data Structures
Additionally, when working with complicated data structures like arrays or objects, where the number of items may change depending on user input, dynamically adding and removing form fields may be required. When creating forms for e-commerce websites or other applications where customers would need to submit many items or details, this might be quite helpful. This is true regardless of whether you're using React in Laravel 9 or React alone for your web development, as both frameworks can benefit from this functionality. However, if you're specifically comparing Laravel vs React, the choice between these two technologies depends on your specific use case and development needs.
Make Forms Flexible and Easier to use
On the whole, dynamically adding and removing form fields is an effective technique that can enhance the user experience, make forms more flexible, and make them simpler to use. Regardless of how complex your data structures are or if you are looking at Laravel vs React, you can simply integrate this feature into your web apps by using React and React Hooks. If you are using Laravel 9, integrating React can be a powerful addition to your toolkit, allowing you to build highly interactive and dynamic interfaces with ease. By using Laravel 9 with React, you can take advantage of the powerful features of both frameworks and create sophisticated, robust web applications that meet your users' needs.
Step 1: Create a form with inputs for name and email
In the above code, you can see how the React useState hook with an array is used to set default values, which is an important feature when building dynamic forms with React in Laravel 9. Besides this, the map() method is utilized to iterate state elements so that the process of adding and removing form fields is much more efficient and user-friendly.
Step 2: Add functions to create add and remove fields
handleChange: This function is used to set input field values based on an index and name on Change. This function is an essential part of our dynamic form implementation using React in Laravel 9. It allows us to update the values of input fields dynamically based on user interactions, making the form-filling process smoother and more intuitive. By using this function in conjunction with other React Hooks and components, we can create dynamic forms that are both efficient and user-friendly.
addFormFields: In this step, we'll create functions to add and remove form fields dynamically. The addFormFields method makes it easy to add a new element with input fields, which is where the power of React in Laravel 9 comes into play.
removeFormFields: To remove elements based on an index.
Complete Example with Function Component
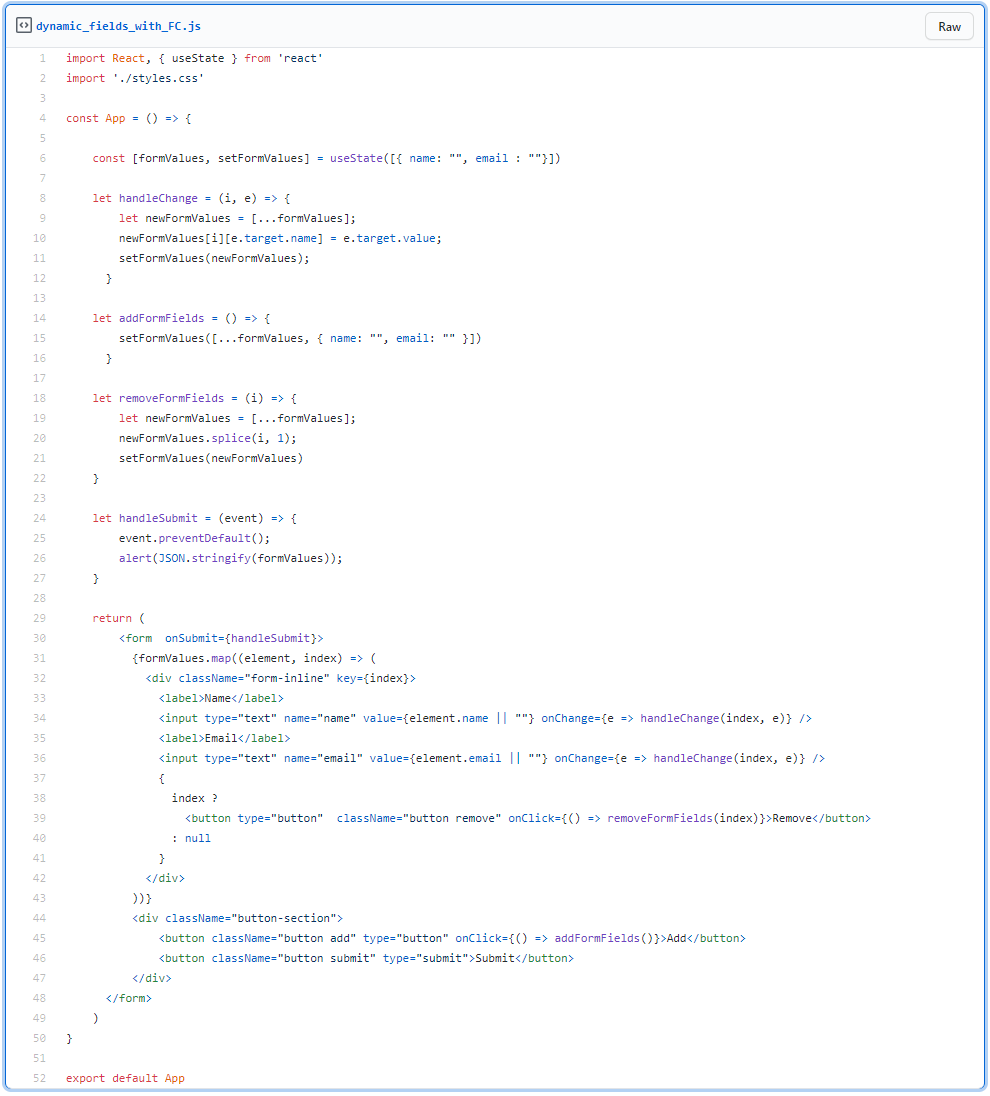
Complete Example with Class component
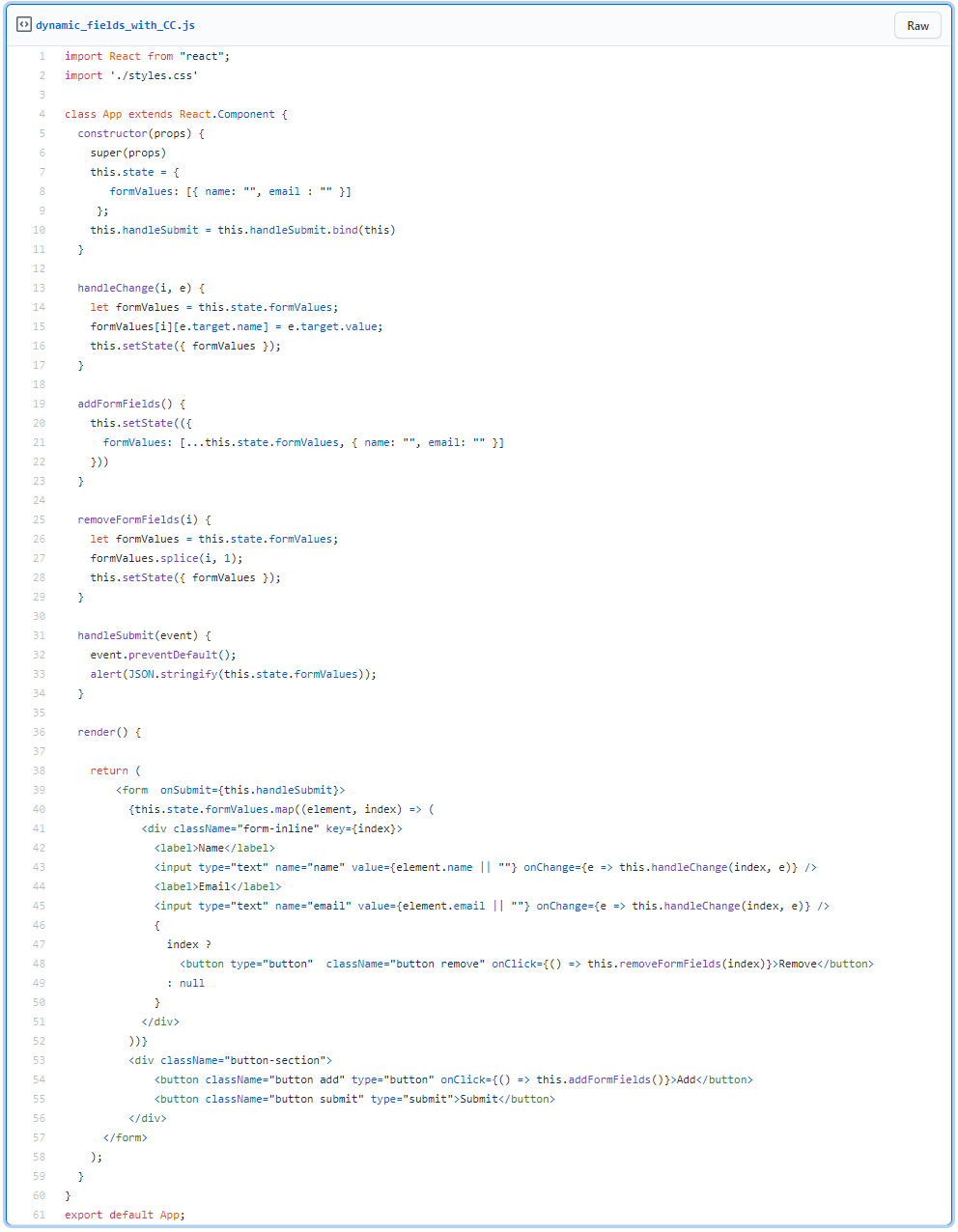
CSS code
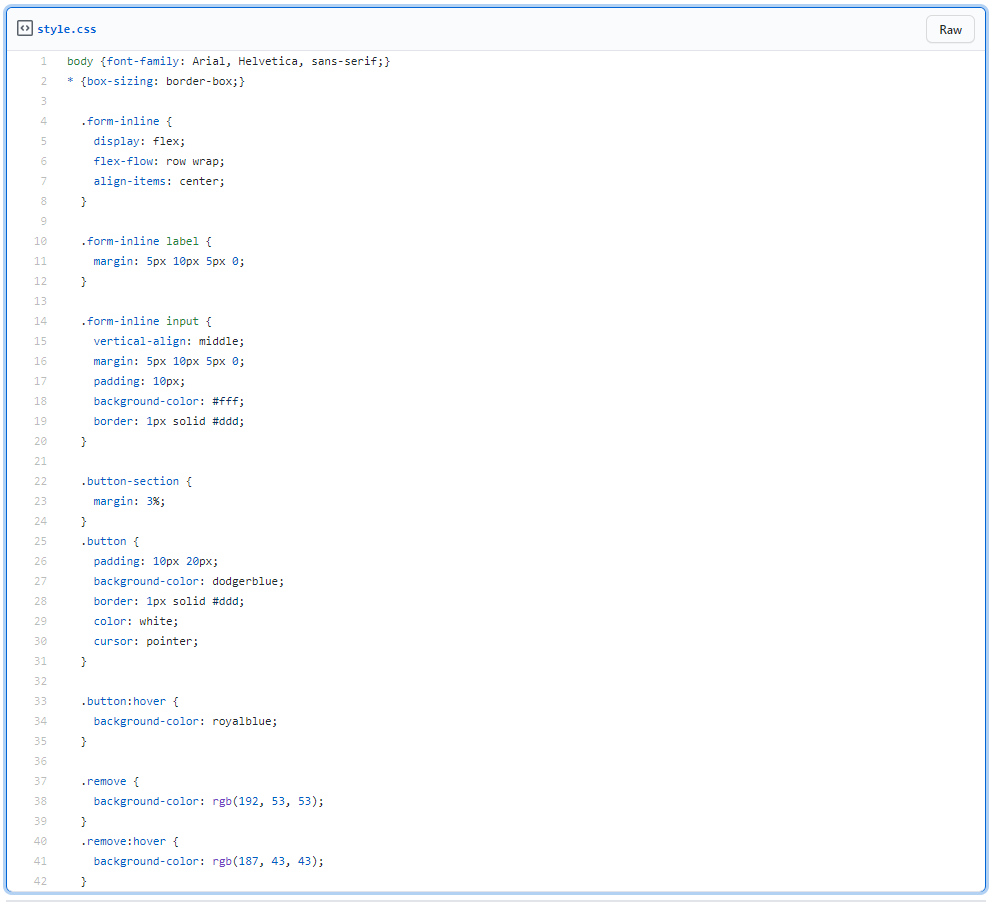
Output
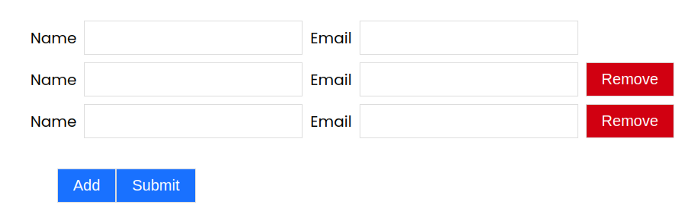
In conclusion, Laravel 9's React and React Hooks are excellent tools for creating dynamic web forms, and improving the usability and intuitiveness of the form-filling process. You can quickly discover how to use these technologies to develop efficient forms by paying attention to the instructions provided in this tutorial. However, developing high-quality software requires more than just implementing the latest technologies. So ensure your software meets the highest standards of quality and reliability by partnering with a reputable UAT Tester.
When comparing Laravel vs React, React is a JavaScript library used for creating user interfaces, while Laravel is a PHP framework that offers a rich set of tools for server-side web development. Although each has benefits and drawbacks, Laravel 9 and React can be combined to create a web development stack that is strong and effective.
For developing this dynamic form logic utilizing both class and functional components, we've provided the entire code. By using the React useState hook, map() method, and other components, you can create dynamic forms that are both effective and simple to use.
FAQs:
1. What are dynamic form fields?
Ans: Dynamic form fields are form fields that can be added or removed dynamically by users as needed, rather than having a fixed number of fields.
2. Why is it useful to dynamically add and remove form fields?
Ans: Dynamically adding and removing form fields can make the form more user-friendly and versatile, streamline the user input process, and help when dealing with complex data structures.
3. What is the advantage of using React in Laravel 9 to create dynamic form fields?
Ans: Using React in Laravel 9 to create dynamic form fields can help developers create robust web applications that are flexible and easy to use, with scalable and secure backends using Laravel and dynamic, responsive user interfaces with React.
4. How can developers create dynamic form fields using React and React Hooks?
Ans: Developers can use the React useState hook with an array to set default values, the map() method to iterate state elements, and functions such as handleChange, addFormFields, and removeFormFields to dynamically update and manage form fields.
5. Can dynamic form fields be implemented with class components in React?
Ans: Yes, dynamic form fields can be implemented using class components in React, and the tutorial provides the entire code to implement this logic using class components.
All in all, using React with Laravel 9 to develop dynamic forms is wise and useful for both personal and business web projects. So go ahead and give it a try!
If you are looking for the best web development company in New York, we can help. Contact us today!
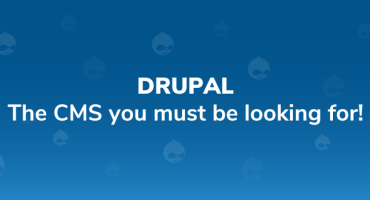
DRUPAL: The CMS you must be looking for!
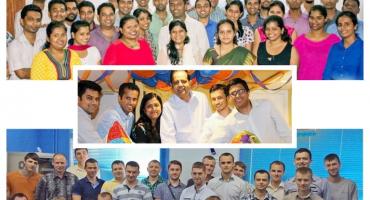
Culture & happiness in SJI
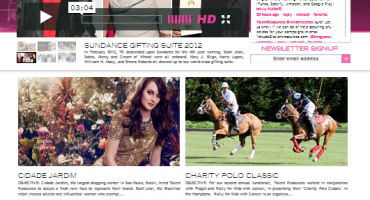