Securing PHP Applications: A Comprehensive Guide
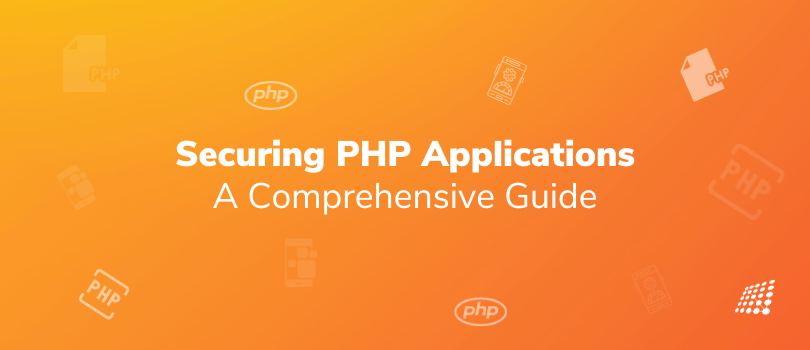
One of the most popular server-side programming languages for developing websites is PHP. However, because of its prominence, attackers also find it to be a desirable target. So, to safeguard confidential information and preserve the integrity of your online projects, you must secure PHP apps!
How to secure a PHP website and application, you ask? We will cover every facet of PHP application security in this in-depth book, from server and network security to code-level security techniques. You should have a firm grasp of how to secure a PHP website and how to protect your PHP apps against common vulnerabilities by the time you finish reading this article.
Table of Contents
- Introduction
- The Importance of PHP Application Security
- Common PHP Security Threats
- Code-Level Security
- Input Validation and Sanitization
- SQL Injection Prevention
- Cross-Site Scripting (XSS) Mitigation
- Cross-Site Request Forgery (CSRF) Protection
- Secure File Uploads
- Avoiding Code Injection
- Session Management and Cookies
- Secure Communication
- Using HTTPS
- SSL/TLS Configuration
- HTTP Security Headers
- Data Encryption
- Authentication and Authorization
- Password Hashing
- Multi-Factor Authentication (MFA)
- Role-Based Access Control (RBAC)
- User Session Management
- Server and Network Security
- Web Server Hardening
- PHP Configuration
- File and Directory Permissions
- Firewall Setup
- Database Security
- Secure Database Connections
- SQL Injection Prevention
- Database User Privileges
- Secure File Handling
- File Uploads
- File Inclusion Vulnerabilities
- Directory Traversal
- Third-Party Libraries and Dependencies
- Keeping Libraries Up-to-Date
- Secure Coding Practices for Libraries
- Error Handling and Logging
- Handling Errors Safely
- Appropriate Logging Practices
- Security Testing and Auditing
- Automated Scanning Tools
- Manual Code Review
- Penetration Testing
- Security Best Practices in PHP Frameworks
- Laravel
- Symfony
- CodeIgniter
- Additional Security Measures
- Content Security Policy (CSP)
- Clickjacking Protection
- Security Headers
- Incident Response and Recovery
- Preparing for Security Incidents
- Responding to Security Breaches
- Conclusion
- The Ever-Evolving Landscape of PHP Security
- Resources for Further Learning
1. Introduction
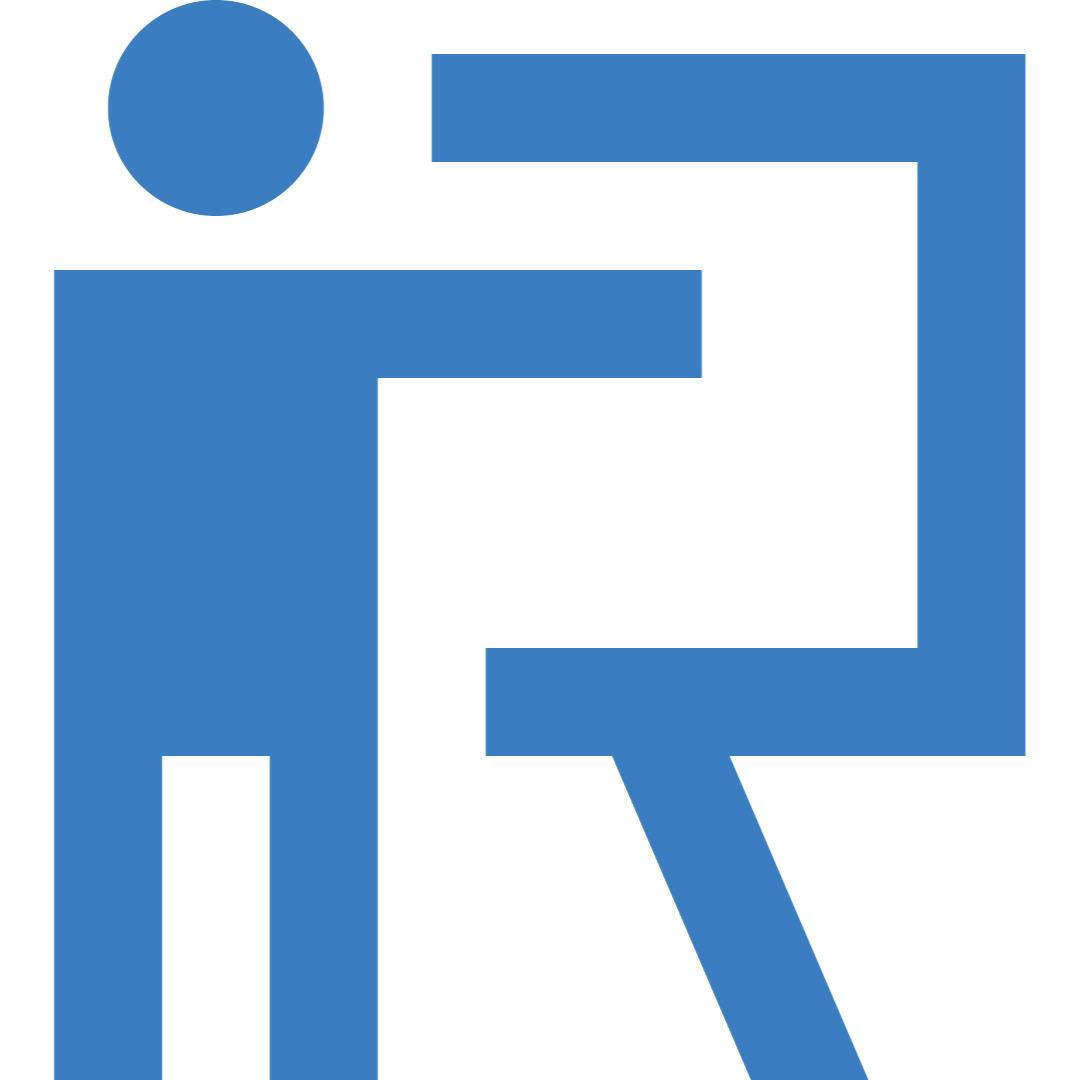
The Importance of PHP Application Security
PHP is well-known for being flexible, user-friendly, and having a vibrant development community. If developers are careless, these same characteristics might potentially result in security flaws. Data leaks, monetary losses, and reputational harm to a company can all be caused by security breaches.
It's not just a smart practice but also a must to make sure your PHP apps are secure. Adopting strong security measures will protect your apps from attacks such as data leaks, cross-site scripting, and SQL injection.
The role of PHP (Hypertext Preprocessor) is to primarily drive web development, enabling the creation and management of dynamic web pages, content management systems, and e-commerce applications.
- It facilitates interaction with databases, such as MySQL, to store, retrieve, and manipulate data, which is essential for building data-driven websites.
- Moreover, PHP offers a wide array of functions and frameworks, like Laravel, to streamline backend development, improve security, and accelerate the time-to-market for web-based solutions.
Common PHP Security Threats
It's important to comprehend the typical security threats in PHP before delving into security best practices:
a. SQL Injection (SQLi)
When malicious code is injected into SQL queries, an attacker can use various SQL injection payloads to manipulate the database operations, potentially modifying the results or executing unintended actions. This is known as SQL injection, a critical vulnerability that can lead to serious security breaches, including data theft and unauthorized database access. By exploiting such vulnerabilities, attackers may gain the ability to read sensitive data, alter or destroy it, or gain administrative access to the database system.
b. Cross-Site Scripting (XSS)
XSS attacks, specifically reflected cross-site scripting, occur when an attacker sends a malicious script as part of an HTTP request, which is then executed by the victim's browser as if it were part of the target website. This type of XSS attack entails inserting harmful scripts into other users' websites that are immediately returned in the website's response, often through error messages or search results. Such vulnerabilities can lead to the theft of user data, session hijacking, and other significant security compromises when the user's browser executes the script without realizing its malicious intent.
c. Cross-Site Request Forgery (CSRF)
CSRF attacks, which exploit a server-side request forgery vulnerability, deceive users into performing unwanted actions on a website where they're currently authenticated. For example, an attacker could craft a malicious request to exploit this vulnerability and orchestrate a scenario where, without the user's knowledge, actions such as changing the user's password are carried out. This can occur when a web application fails to properly verify whether a request was intentionally made by the authenticated user, thereby allowing attackers to trick the client-side browser into sending forged requests to the server.
d. File Upload Vulnerabilities
If user files are not adequately vetted, attackers could exploit a file upload vulnerability by uploading malicious files, potentially compromising users' security and jeopardizing the integrity of the web application. An example of such a vulnerability is when an attacker is able to upload a script disguised as an image file, and the server mistakenly executes it, granting the attacker the ability to take control of the server or perform other unauthorized activities. This underscores the importance of implementing stringent upload controls and file validation checks to prevent the exploitation of file upload vulnerabilities.
e. Insecure Session Management
Session hijacking, which enables attackers to assume user identities, can be caused by inadequate session management.
After discussing the overview, let's go into the specifics of PHP application security.
2. Code-Level Security
Input Validation vs Sanitization
Input validation and sanitization are a PHP application's first line of defense. To make sure they meet the intended format and range, all user input should be carefully checked. To remove possibly harmful characters or data, sanitization should also be used.
$userInput = $_POST['username'];
$cleanedInput = filter_var($userInput, FILTER_SANITIZE_STRING);
SQL Injection Prevention
One crucial component of PHP application security is preventing SQL injection. The best defenses against SQL injection are prepared statements and parameterized queries.
// Using PDO prepared statements
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username");
$stmt->bindParam(':username', $username);
$stmt->execute();
Cross-Site Scripting (XSS) Mitigation
By verifying and escaping output data, XSS attacks may be prevented. Content created by users should have its HTML and JavaScript encoded or cleaned up.
$unsafeData = $_GET['message'];
$cleanData = htmlspecialchars($unsafeData, ENT_QUOTES, 'UTF-8');
Cross-Site Request Forgery (CSRF) Protection
Forms that use anti-CSRF tokens can stop CSRF attacks. These tokens need to be supplied with every request as they are unique to each user session.
// Generating and including CSRF token
$token = bin2hex(random_bytes(32));
$_SESSION['csrf_token'] = $token;
Secure File Uploads
How to send a secure file? Make sure that only certain file kinds and secure file names are accepted if file uploads are permitted. To further limit direct access, store uploaded files somewhere other than the site root.
// Check file type and move to safe location
if (in_array($fileType, $allowedTypes)) {
move_uploaded_file($_FILES['file']['tmp_name'], '/path/to/uploads/' . $newFileName);
}
Avoiding Code Injection
Do not run input from the user as code. Code injection vulnerabilities may arise from this method.
// Unsafe code execution
eval($_POST['user_code']);
Session Management and Cookies
Understand session management vulnerabilities and make use of secure session management techniques, such as session timeouts, secure session regeneration, and secure session cookies.
// Setting secure session cookie options
session_set_cookie_params([
'secure' => true,
'httponly' => true,
]);
3. Secure Communication
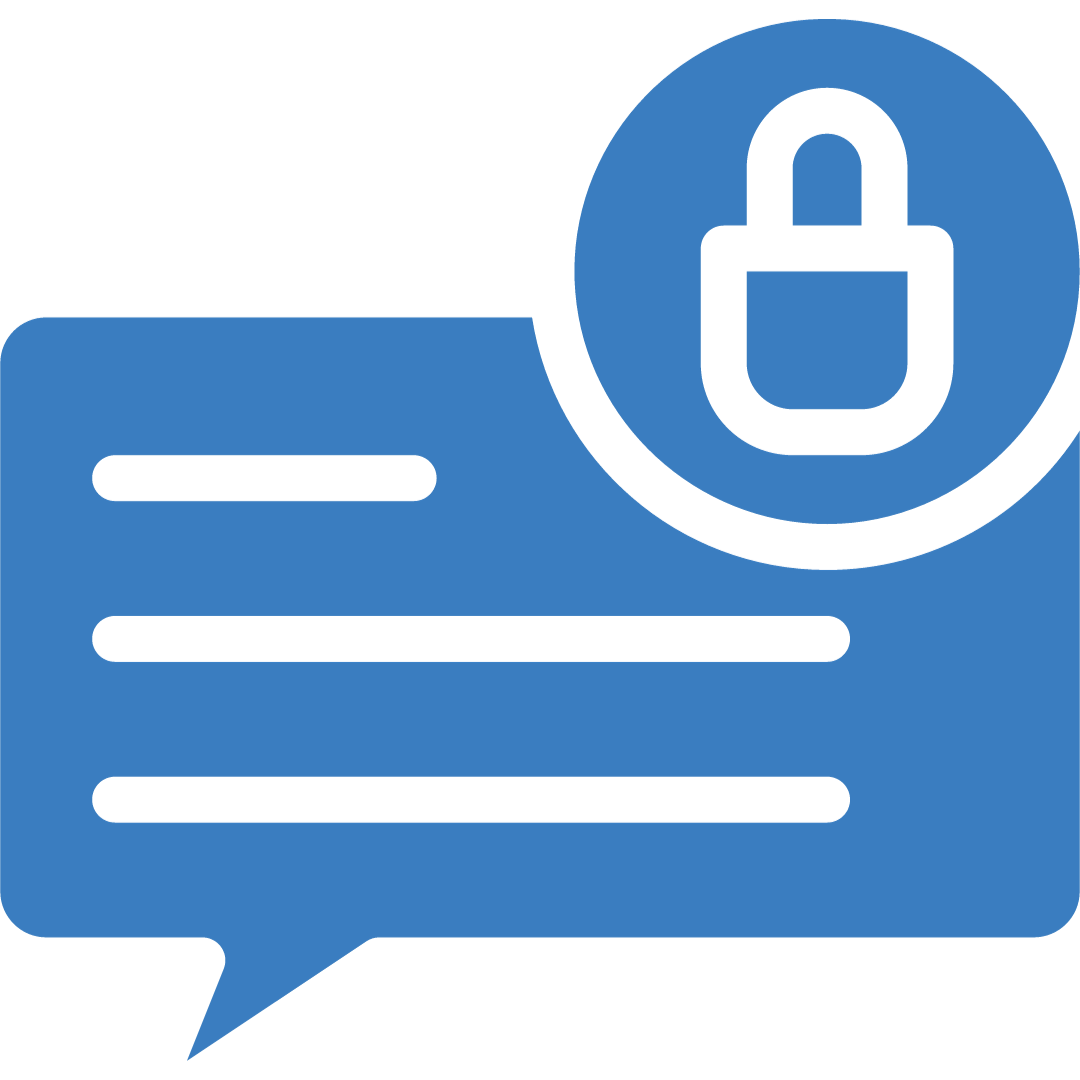
Using HTTPS
HTTPS should be used for all client-server communication. To encrypt data while it is in transit, SSL certificates are necessary.
SSL/TLS Configuration
Using robust ciphers, protecting key and certificate files, and maintaining server software updates are all part of proper SSL/TLS configuration.
HTTP Security Headers
To reduce the possibility of Clickjacking and Cross-Origin Resource Sharing (CORS), among other web application security vulnerabilities, add security headers to your web server settings.
Header always set Content-Security-Policy "default-src 'self';"
Data Encryption
Databases holding sensitive information have to be encrypted. For at-rest data protection, use encryption libraries.
// Encrypting data before storage
$encryptedData = openssl_encrypt($data, 'aes-256-cbc', $encryptionKey, 0, $iv);
4. Authentication and Authorization
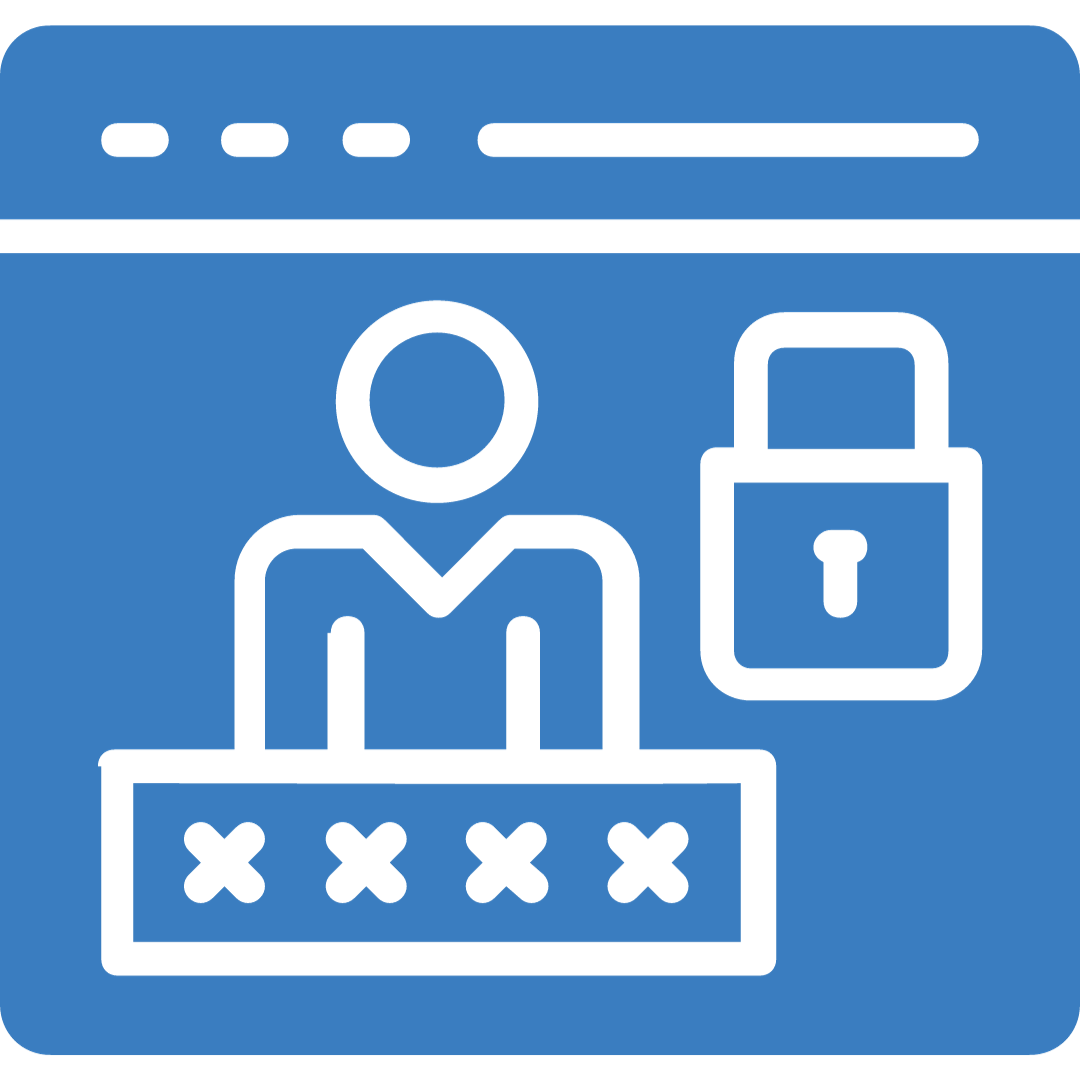
Password Hashing
Prevent storing passwords in plain text. Passwords should be hashed and verified using secure hashing methods like bcrypt.
// Hashing a password
$hashedPassword = password_hash($password, PASSWORD_BCRYPT);
Multi-Factor Authentication (MFA)
Use MFA to provide user accounts an additional degree of protection. Passwords and user-possessed items—such as mobile apps or hardware tokens—are usually involved in this.
Role-Based Access Control (RBAC)
To control user access privileges, utilize RBAC. To restrict what users can and cannot do within the program, assign roles and permissions.
// Checking user role for authorization
if ($user->hasPermission('edit_post')) {
// Allow editing
}
User Session Management
Make sure user sessions are handled safely. Integrate functions such as the automated expiry of sessions following a period of inactivity.
5. Server and Network Security
Web Server Hardening
Use a Web Application Firewall (WAF), apply frequent security updates, and disable superfluous services to safeguard your web server.
PHP Configuration
To reduce possible attack vectors, review your PHP settings. Disable risky functions and configure PHP according to the necessary security requirements.
// Disabling potentially dangerous functions
disable_functions = exec, system, shell_exec, passthru
File and Directory Permissions
To guarantee that only authorized users may access and alter data, set stringent permissions for directories and files.
# Example of setting file permissions
chmod 644 /path/to/sensitive-file.php
Firewall Setup
Installing a firewall will shield your server against DDoS assaults and illegal access. Set it up to only let through essential traffic.
# Setting up a basic firewall rule
iptables -A INPUT -p tcp --dport 80 -j DROP
6. Database Security
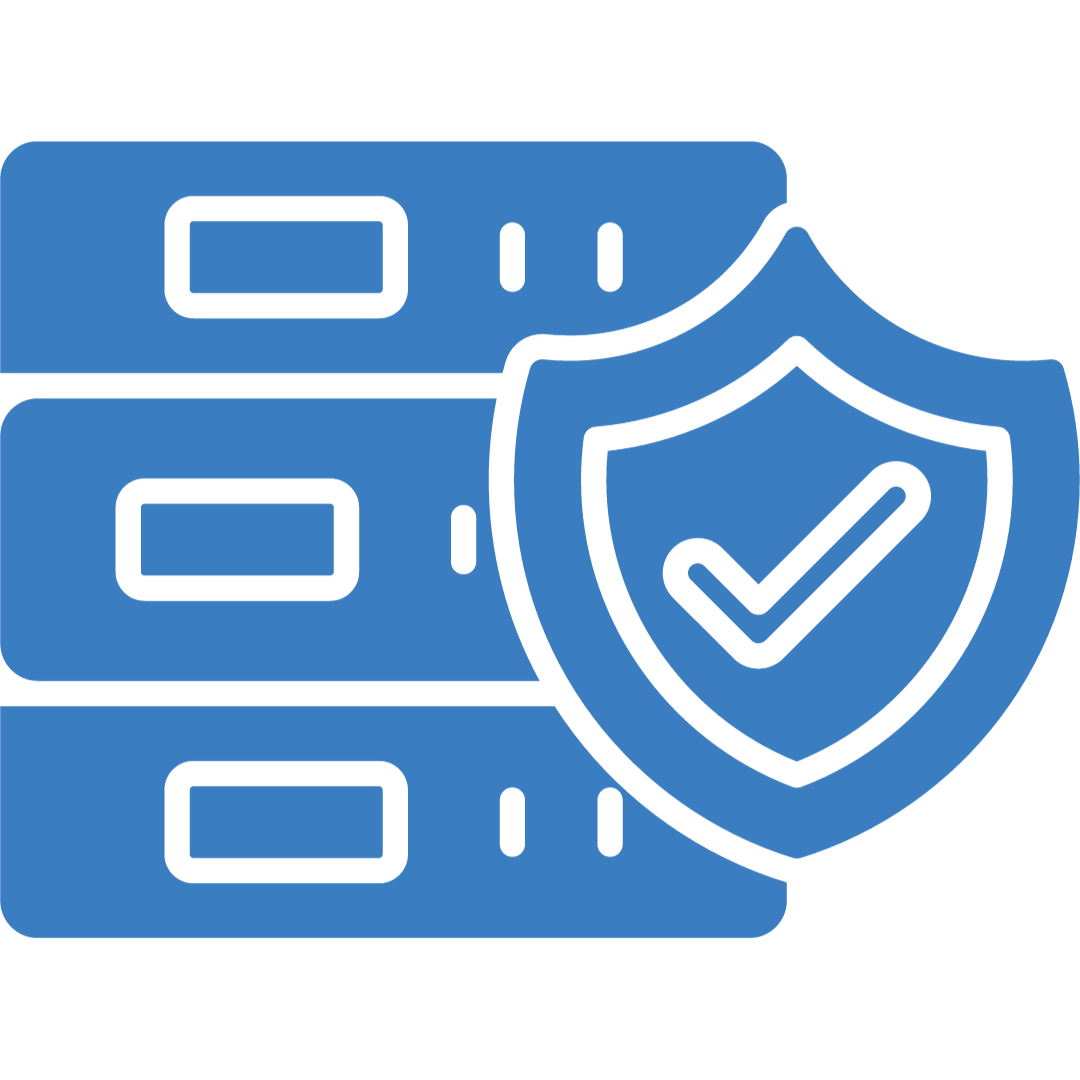
Secure Database Connections
To encrypt communication between your PHP application and the database, use secure connection techniques like SSL.
SQL Injection Prevention
Although we've talked about this before, it bears repeating. To stop SQL injection, use prepared statements and parameterized queries.
Database User Privileges
Give database users the bare minimum of rights. Don't connect to databases using a superuser account.
7. Secure File Handling
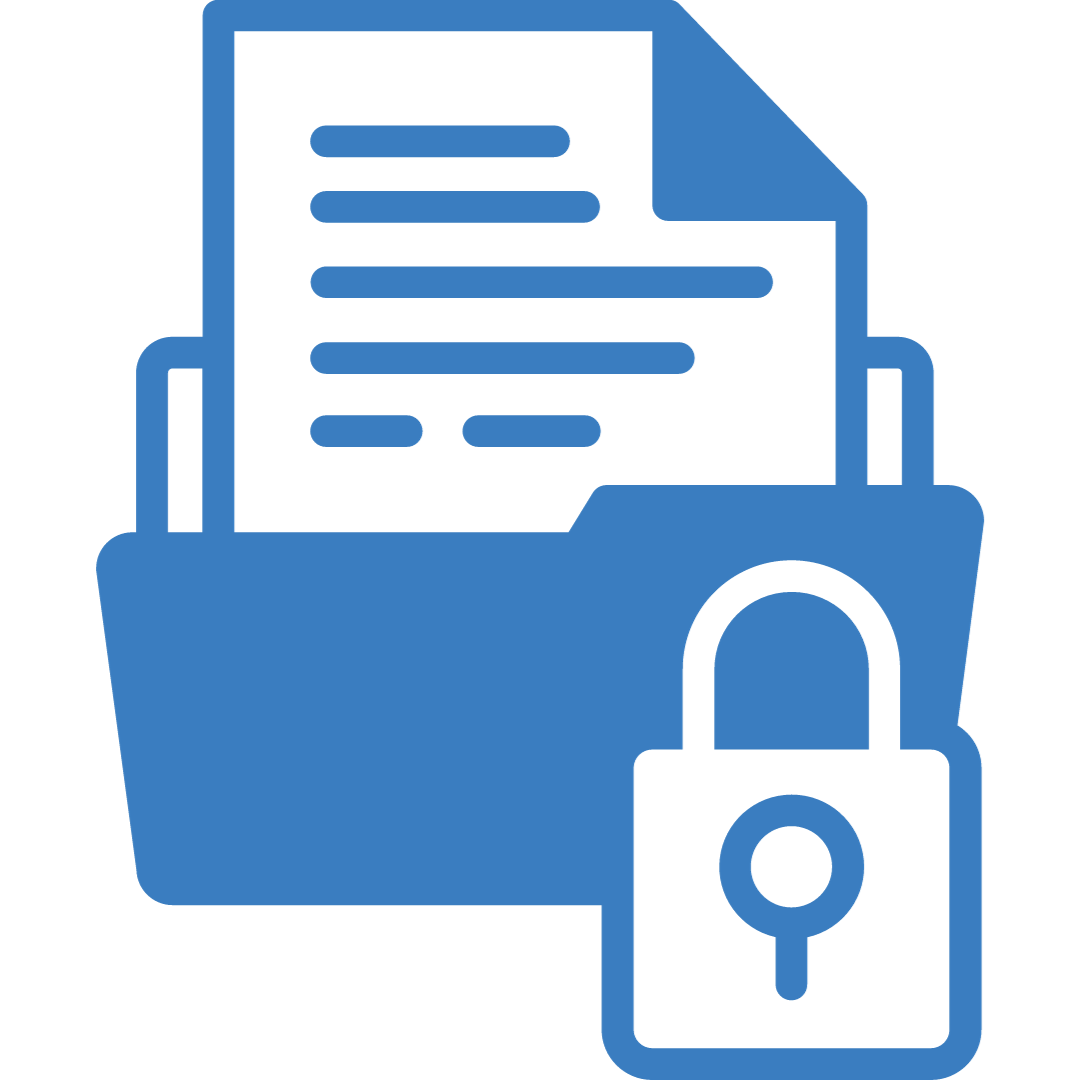
File Uploads
Verify file types and make sure submitted files are not executable. Keep uploaded files off of the main web directory.
File Inclusion Vulnerabilities
Don't utilize user input when including files. To designate acceptable files, use a whitelist technique.
Directory Traversal
By cleaning up file paths and avoiding using user-controlled routes for filesystem operations, you can stop directory traversal threats.
8. Third-Party Libraries and Dependencies
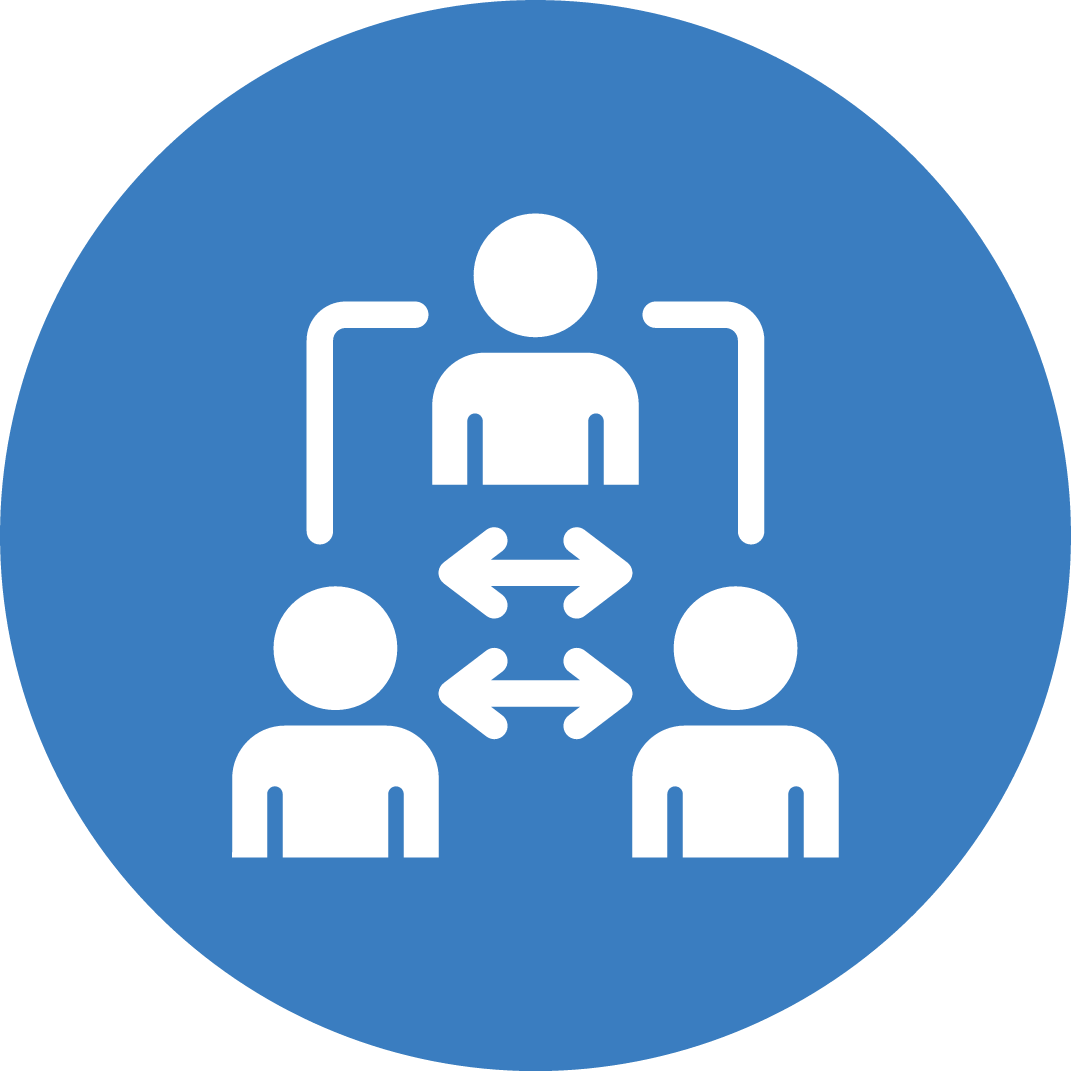
Keeping Libraries Up-to-Date
Update dependencies and third-party libraries often to fix security flaws. Handle dependencies with tools such as Composer.
Secure Coding Practices for Libraries
Make sure you validate inputs following third-party libraries' documentation and adhere to their security best practices while using them.
9. Error Handling and Logging
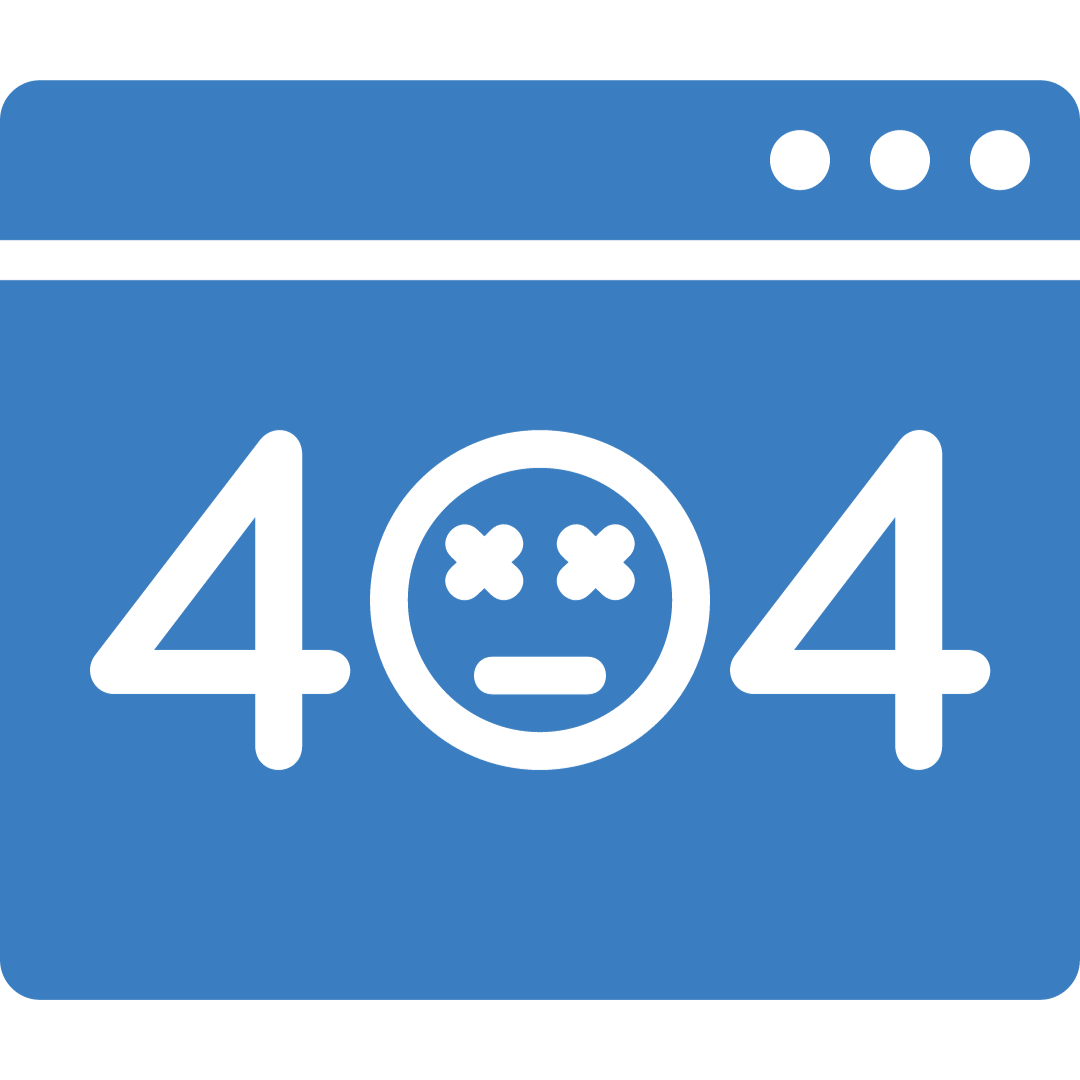
Handling Errors Safely
Do not provide end users with comprehensive error messages. Enable the program to gracefully handle failures and securely log them.
Appropriate Logging Practices
Keep track of security-related events, such as login attempts, so you can keep an eye out for unusual activity. Logs should be safely stored and periodically examined.
10. Security Testing and Auditing
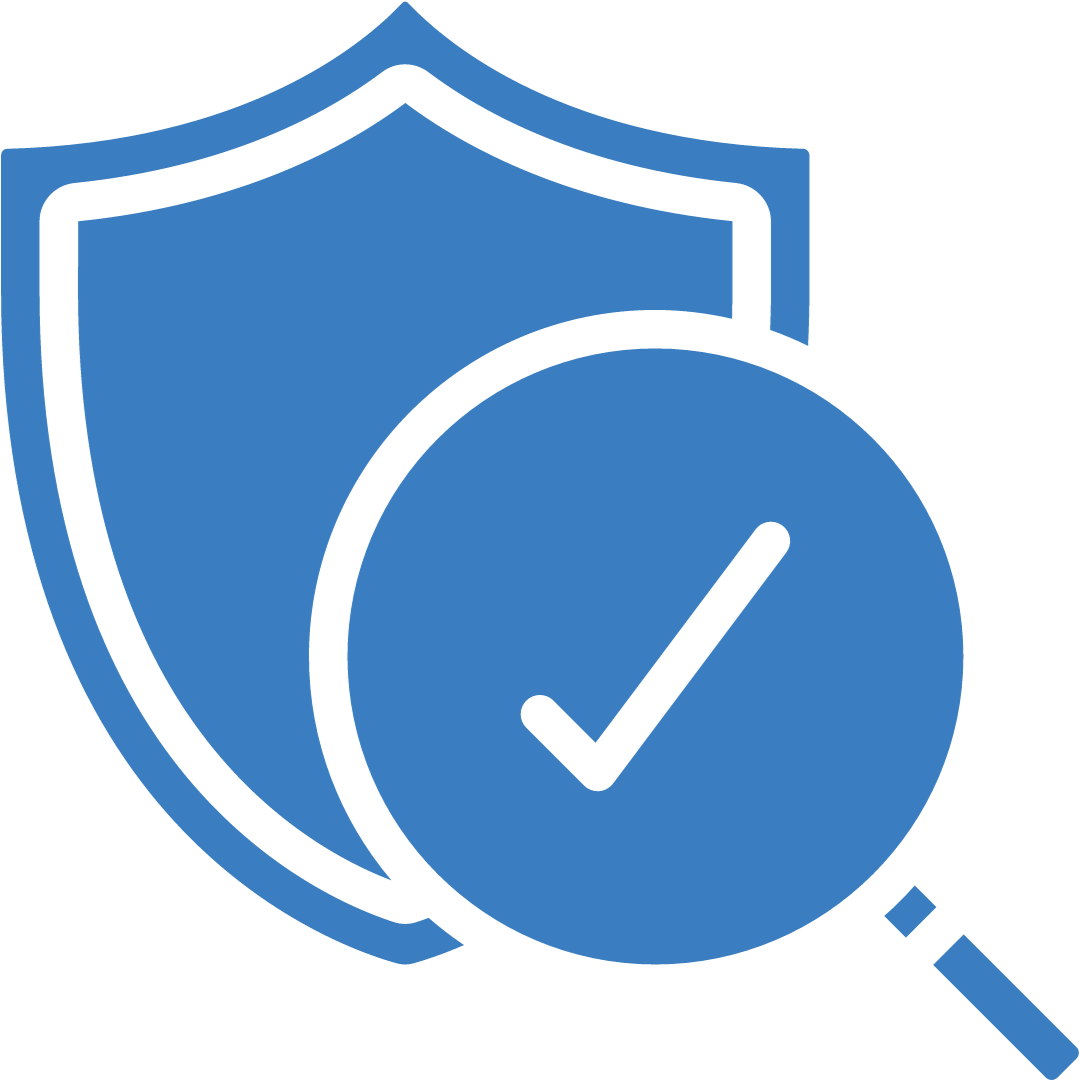
Automated Scanning Tools
For PHP applications, use automated security scanning tools such as Nessus, Qualys, or OWASP ZAP to find vulnerabilities.
Manual Code Review
Review the code manually to find security flaws that automated tools might overlook. This is particularly crucial for the application's crucial sections.
Penetration Testing
To replicate real-world assaults and find weaknesses in your application, hire a professional penetration testing team.
11. Security Best Practices in PHP Frameworks
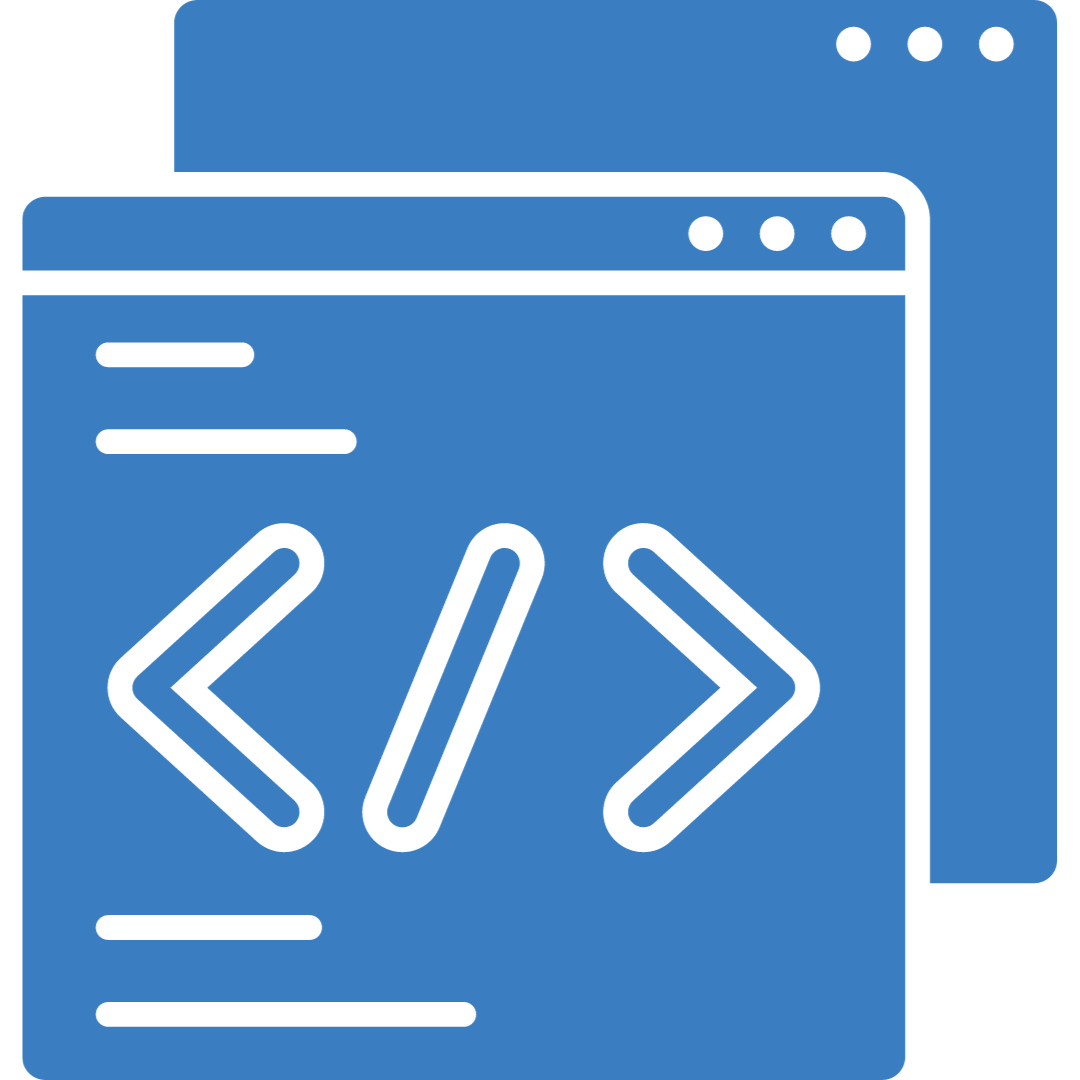
Laravel
- Use the integrated authentication mechanism in Laravel.
- CSRF protection may be implemented using @csrf tokens.
- Use Eloquent ORM to operate databases securely.
Symfony
- To enable access control, configure security.yaml.
- Use the Symfony Security module to provide access and authenticate users.
- Use user-controlled inputs with caution.
CodeIgniter
- Utilize the XSS filtering and other built-in security capabilities of CodeIgniter.
- Make CSRF prevention active by default.
- Take advantage of form validation rules to verify user input.
12. Additional Security Measures
Content Security Policy (CSP)
To limit the sources of scripts and material that may be run on your websites, implement a content security policy.
Clickjacking Protection
By configuring the X-Frame-Options headers to refuse framing, you can defend against clickjacking assaults.
Header always append X-Frame-Options SAMEORIGIN
Security Headers
To increase security, set different security headers such as X-XSS-Protection, X-Content-Type-Options, and Referrer-Policy.
13. Incident Response and Recovery
Preparing for Security Incidents
Create an incident response plan outlining the actions to be taken in the event of a security breach.
Responding to Security Breaches
In the event of a security breach, take the appropriate action as outlined in your incident response plan, notify the parties involved, and investigate the reason.
14. Conclusion
Application security for PHP is a continuous process. Your security procedures should change along with the threat landscape. Keep up with new developments in the field, keep up with potential threats, and keep an eye out for security holes in your apps.
Keep in mind that developers, system administrators, and users all work together to provide security. You may drastically lower the chance of security breaches in your PHP apps by paying close attention to the recommended practices described in this article and exercising caution.
Resources for Further Learning
In summary, protecting PHP applications requires a variety of techniques, including secure communication, server and network security, code-level security, authentication, and permission. Your PHP apps' security may be greatly improved, and you can keep them safe from common attacks, by implementing the best practices and advice described in this book. Maintain the latest versions of your programs and dependencies, be proactive in your security activities, and be ready to react quickly to security issues.
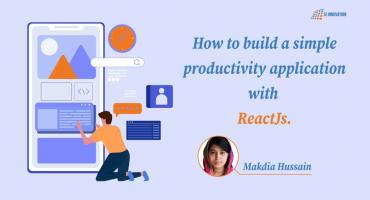
How To Build A Simple Productivity Application With ReactJs
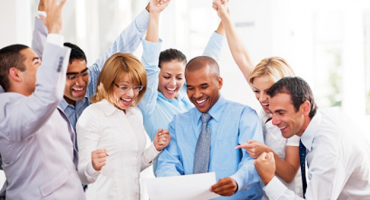
Significance of positive work environment
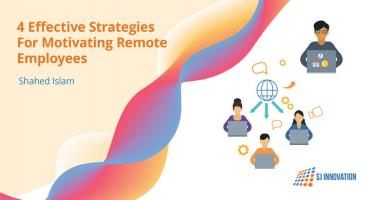